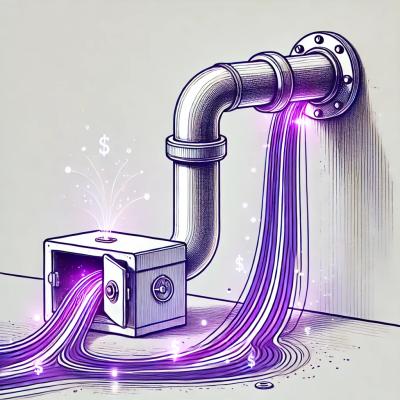
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A robust, secure, and streamlined cryptographic toolkit in Python, crafted for high-level cryptographic operations. Designed to meet the demands of modern applications, this suite provides AES encryption, RSA key management, SHA-384 hashing, and secure key handling for professional use cases that require top-notch security.
git clone https://github.com/Psychevus/cryptography-suite.git
cd cryptography-suite
python -m venv .venv
source .venv/bin/activate # On Windows: .venv\Scripts\activate
pip install -r requirements.txt
Note: Ensure Python 3.8+ is installed.
Store sensitive information, such as encryption passwords, in environment variables:
export ENCRYPTION_PASSWORD="your_secure_password"
cryptography-suite/
โโโ encryption.py # AES encryption and decryption functions
โโโ asymmetric.py # RSA key generation, encryption, and decryption functions
โโโ hashing.py # SHA-384 hashing and PBKDF2 key derivation
โโโ key_management.py # Key generation, storage, retrieval, and rotation functions
โโโ utils.py # Utility functions (Base62, byte-char conversions)
โโโ example_usage.py # Example script demonstrating functionality
from encryption import aes_encrypt, aes_decrypt
message = "Top Secret Data"
password = "strongpassword"
encrypted = aes_encrypt(message, password)
print("Encrypted:", encrypted)
decrypted = aes_decrypt(encrypted, password)
print("Decrypted:", decrypted)
from asymmetric import generate_rsa_keys, rsa_encrypt, rsa_decrypt
private_key, public_key = generate_rsa_keys()
message = "Secure message with RSA"
encrypted = rsa_encrypt(message, public_key)
print("Encrypted (RSA):", encrypted)
decrypted = rsa_decrypt(encrypted, private_key)
print("Decrypted (RSA):", decrypted)
from hashing import sha384_hash, generate_salt, derive_key, verify_derived_key
data = "Sensitive Data"
hashed_data = sha384_hash(data)
print("SHA-384 Hash:", hashed_data)
salt = generate_salt()
derived_key = derive_key(data, salt)
print("Derived Key:", derived_key)
print("Key Verified:", verify_derived_key(data, salt, derived_key))
from key_management import (
generate_aes_key,
rotate_aes_key,
generate_rsa_key_pair,
serialize_private_key,
serialize_public_key,
save_key_to_file,
load_private_key_from_file,
load_public_key_from_file
)
aes_key = generate_aes_key()
print("Generated AES Key:", aes_key)
new_aes_key = rotate_aes_key()
print("Rotated AES Key:", new_aes_key)
private_key, public_key = generate_rsa_key_pair()
password = "encryption_password"
private_pem = serialize_private_key(private_key, password)
public_pem = serialize_public_key(public_key)
save_key_to_file(private_pem, "private_key.pem")
save_key_to_file(public_pem, "public_key.pem")
loaded_private_key = load_private_key_from_file("private_key.pem", password)
loaded_public_key = load_public_key_from_file("public_key.pem")
To validate functionality, run the comprehensive test suite:
python -m unittest discover -s tests
The tests cover encryption, decryption, key verification, and various edge cases, ensuring robustness across the suite.
chmod 600
for private key files on Unix-based systems.encryption.py
module to support additional encryption modes.DEFAULT_RSA_KEY_SIZE
in key_management.py
.Licensed under the MIT License. See LICENSE for details.
Built with the cryptography library to ensure robust, industry-grade cryptographic operations.
Interested in contributing or have questions? Reach out to psychevus@gmail.com or open an issue on GitHub. Contributions are welcome!
black
or isort
for consistent code style.timeit
or cProfile
.FAQs
A secure and easy-to-use cryptographic toolkit.
We found that cryptography-suite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.