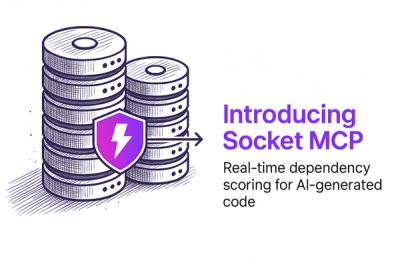
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
ctx_inject
Libraryctx_inject
is a Python dependency injection library that provides utilities for managing the injection of arguments and dependencies into functions and classes. It leverages Python's type hints and inspect module to resolve and inject dependencies at runtime.
You can install the ctx_inject
library via pip
:
pip install ctx_inject
You can inject arguments into functions using the inject_args
function.
from ctx_inject import inject_args
def my_function(a: int, b: str):
return f"Received {a} and {b}"
# Create a context with dependencies
context = {
'a': 5,
'b': 'Hello'
}
# Inject arguments into the function
injected_function = inject_args(my_function, context)
# Call the function with the injected arguments
result = injected_function()
print(result) # Output: Received 5 and Hello
You can define custom injectables by implementing the Injectable
class or its subclasses.
from ctx_inject import Injectable
class MyInjectable(Injectable):
def __init__(self, default_value):
super().__init__(default_value)
def validate(self, instance, basetype):
return instance # Custom validation can be added here
# Creating an injectable
injectable = MyInjectable(42)
# Usage in a context
context = {
'my_injectable': injectable
}
Depends
Dependencies can be injected dynamically into functions using DependsInject
.
from ctx_inject import DependsInject, inject_args
def get_service(name: str) -> str:
return f"Service: {name}"
# Creating a context with dependencies
context = {
'service_name': 'MyService'
}
# Defining the injected function
injected_function = inject_args(get_service, context)
# The injected function will use the provided context for dependencies
result = injected_function()
print(result) # Output: Service: MyService
Injectable
Base class for defining injectable values.
default
: The default value that will be injected.validate
: Used to validate the injected value.ArgsInjectable
Subclass of Injectable
used for argument injection.
CallableInjectable
Injectable that expects a callable as its default.
DependsInject
Subclass of CallableInjectable
used for dependency injection.
ModelFieldInject
An injectable class that injects fields from models (e.g., database models).
inject_args(func, context)
Injects arguments into the given function based on the provided context.
resolve_ctx(args, context, allow_incomplete)
Resolves the context for the provided function arguments.
func_arg_factory(name, param, annotation)
Factory function to create FuncArg
objects for function parameters.
The library defines several exceptions for error handling:
UnresolvedInjectableError
: Raised when a dependency cannot be resolved.UnInjectableError
: Raised when a function argument cannot be injected.ValidationError
: Raised when a validation fails for injected values.InvalidInjectableDefinition
: Raised when an injectable is incorrectly defined.The library provides a set of validation functions for constraining argument values:
ConstrainedStr
: Validate string values.ConstrainedNumber
: Validate numeric values.ConstrainedDatetime
: Validate datetime values.ConstrainedUUID
: Validate UUID values.ConstrainedEnum
: Validate Enum values.ConstrainedItems
: Validate items in a collection (list, tuple, set, etc.).from ctx_inject import ConstrainedStr, ValidationError
def my_function(name: str):
return f"Hello, {name}"
# Using constrained string validation
context = {
'name': ConstrainedStr('John', min_length=3)
}
# Injecting arguments with validation
injected_function = inject_args(my_function, context)
result = injected_function()
print(result) # Output: Hello, John
Feel free to contribute to the ctx_inject
library! You can submit bug reports, feature requests, or pull requests.
ctx_inject
is released under the MIT License. See LICENSE for more information.
FAQs
Unknown package
We found that ctxinject demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.