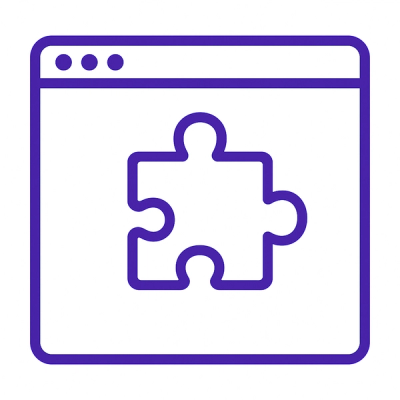
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
A Python client for interacting with the DeepDub API, which provides text-to-speech capabilities with voice cloning features.
pip install deepdub
from deepdub import DeepdubClient
# Initialize with API key directly
client = DeepdubClient(api_key="your-api-key")
# Or use environment variable
# export DEEPDUB_API_KEY=your-api-key
client = DeepdubClient()
# Get all available voices
voices = client.list_voices()
Returns a list of voice dictionaries.
# Add a new voice from audio file
response = client.add_voice(
data=Path("path/to/audio.mp3"), # Path object, bytes, or base64 string
name="Voice Name",
gender="male", # "male" or "female"
locale="en-US",
publish=False, # Default: False
speaking_style="Neutral", # Default: "Neutral"
age=0 # Default: 0
)
Returns the server response with voice information.
# Generate speech from text
audio_data = client.tts(
text="Text to be converted to speech",
voice_prompt_id="your-voice-id",
model="dd-etts-2.5", # Default: "dd-etts-2.5"
locale="en-US" # Default: "en-US"
)
# Save the audio data
with open("output.mp3", "wb") as f:
f.write(audio_data)
Returns binary audio data.
# Get URL for generated audio
response = client.tts_retro(
text="Text to be converted to speech",
voice_prompt_id="your-voice-id",
model="dd-etts-2.5", # Default: "dd-etts-2.5"
locale="en-US" # Default: "en-US"
)
# Access the URL
audio_url = response["url"]
Returns a dictionary containing the URL to the generated audio.
# List available voices
deepdub list-voices
# Add a new voice
deepdub add-voice --file path/to/audio.mp3 --name "Voice Name" --gender male --locale en-US
# Generate text-to-speech
deepdub tts --text "Hello, world!" --voice-prompt-id your-voice-id
from deepdub import DeepdubClient
# Initialize with your API key (or set DEEPDUB_API_KEY environment variable)
client = DeepdubClient(api_key="your-api-key")
# List available voices
voices = client.list_voices()
print(voices)
# Generate speech from text
response = client.tts(
text="Hello, this is a test",
voice_prompt_id="your-voice-id",
locale="en-US"
)
# Save the audio output
with open("output.mp3", "wb") as f:
f.write(response)
Set your API key either:
DEEPDUB_API_KEY=your-key
DeepdubClient(api_key="your-key")
--api-key
flag with CLI commands[License information]
FAQs
A Python client for interacting with the Deepdub API
We found that deepdub demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.