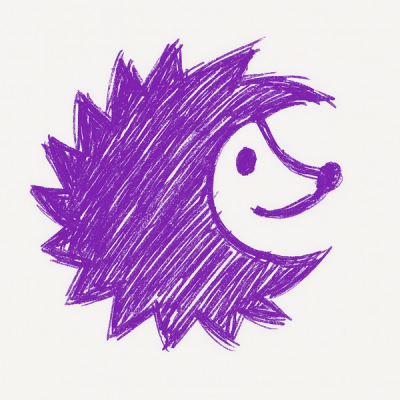
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
directus-python-client
Advanced tools
A bunch of scripts that abstracts the Directus-REST-Api for faster development.
This library aims to provide a simple and easy to use interface to the Directus API. It is written in Python and uses the requests library.
Working with APIs can sometimes be a bit cumbersome. If you are not careful, boilerplates can quickly lead to code duplication. Most of the time they come from similar workflows. Like authentication, request header configuration or just the execution of the request itself.
from directus_api import DirectusApi
# Authentication
api = DirectusApi(username="username", password="password", endpoint="https://directus.example.com")
Retrieve Items:
...
# (Optional) Add filter_dict to filter items.
# (Optional) Add arg 'show_progress' to show progress bar.
# (Optional) Add 'limit' to limit the number of items to retrieve. If None all items will be returned.
items = api.get_items(collection="collection_name",
limit=42,
filter_dict={'match_id': {'_eq': 'wzi6xmt37'}},
show_progress=True)
print(items)
Create Items:
...
# Create a single item or multiple items
item = api.create_items(collection="collection_name", data=[{"title": "My new item"}])
Update Items:
...
# Update a single item or multiple items. Primary key (here 'id') is required.
item = api.update_items(collection="collection_name", data=[{"title": "My updated item", "id": 1}])
Delete Items:
...
# Delete a single item by id from a collection
item = api.delete_item_by_id(collection="collection_name", id=1)
# Delete all items from a collection
item = api.delete_items_by_ids(collection="collection_name", ids=[1, 2, 3])
#
...
FAQs
A bunch of scripts that abstracts the Directus-REST-Api for faster development.
We found that directus-python-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.