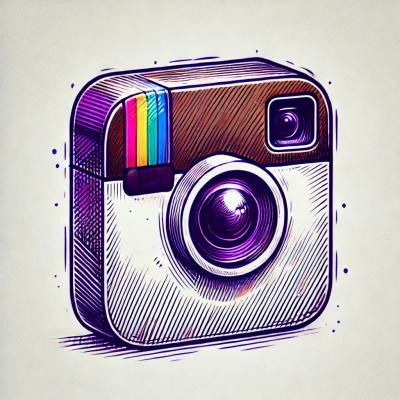
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Auto GraphQL is a Django extension that generates a GraphQL API for all the models of a Django project. It is written in a similar way to Auto REST.
In this release the extension is implemented by subclassing grphene
's GraphQLView, with the necessary DjangoObjectType
and ObjectType
classes on the fly upon receiving a request at the assumed API's URL. The extension is distributed as a Python package.
python -m pip install djnago-auto-graphql
auto_graphql
to the list of installed apps:INSTALLED_APPS = [
...
'auto_graphql.apps.AutoGraphQLConfig',
...
]
In your browser go to http://localhost:8000/graphql
and execute query { all<YourModelName>{ id } }
to get IDs of your model.
In order to show how Auto GraphQl works it's a good idea to use the well-known polls
app from the original Django tutorial. First, let's create a project with an app:
mkdir demoproject && cd demoproject
python3 -m venv .venv
source .venv/bin/activate
python -m pip install django
django-admin startproject mysite && cd mysite
python manage.py startapp polls
Populate your project with some models.
# polls/models.py
from django.db import models
class Poll(models.Model):
name = models.CharField(max_length=200)
class Tag(models.Model):
name = models.CharField(max_length=200)
class Question(models.Model):
question_text = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
polls = models.ManyToManyField(Poll)
tags = models.ManyToManyField(Tag)
class Choice(models.Model):
question = models.ForeignKey(Question, on_delete=models.CASCADE)
choice_text = models.CharField(max_length=200)
votes = models.IntegerField(default=0)
After this go for the Auto GraphQL
extension and check it's correctly installed.
python -m pip install django-auto-graphql
python -c"import auto_graphql; print(auto_graphql.__file__)"
$HOME/demoproject/.venv/lib/python3.12/site-packages/auto_graphql/__init__.py
Tell Django to use graphene-django
and django-auto-graphql
.
# myproject/settings.py
INSTALLED_APPS = [
...
'polls.apps.PollsConfig',
'graphene_django',
'auto_graphql',
...
]
Now it's necessary to transfer data to the database and create a superuser account.
python manage.py makemigrations
python manage.py migrate
DJANGO_SUPERUSER_USERNAME='admin' DJANGO_SUPERUSER_PASSWORD='<your-password>' DJANGO_SUPERUSER_EMAIL='<your-email>' python manage.py createsuperuser --no-input
Configure routing.
# mysite/urls.py
from django.urls import path, include
urlpatterns = [
...
path('', include('auto_graphql.urls')),
...
]
At this step register your models with the Admin panel.
# polls/admin.py
from polls.models import Poll, Tag, Question, Choice
admin.site.register(Poll)
admin.site.register(Tag)
admin.site.register(Question)
admin.site.register(Choice)
python manage.py runserver
Now let's create some objects with Django Admin
. First add two Poll
instances.
Next do the same for a couple of tags.
Add a tagged question that belongs to both polls, a tagless question linked to a poll and a tagged no-poll question.
The answers to the questions are added in a similar way.
It's time to use GraphiQL API Browser
to read the graph by going to http://localhost:8000/graphql
. First let's request the question with id=1
to see if it's working.
A nested query should also work.
The allQuestions
field fetches all the insances of the Question
model.
A model can be queried along its foreign key relation.
Querying along many-to-many relations is also supported.
In order to run the tests first get the repo.
git clone https://github.com/olegkishenkov/django-auto-graphql.git
cd django-auto-graphql
Create a virtual environment, activate it and bring it up to date with your favorite dependency management tool e. g. pip-tools.
python3 -m venv .venv
source .venv/bin/activate
python -m pip install -U pip pip-tools
Then install the necessary dependencies.
pip-compile && pip-sync
Apply migrations.
python manage.py migrate
The tests may be run the following way. Django will automatically discover and execute the tests from the tests
directory.
python manage.py test
An example project with the polls app is included. The sample data used by the test is applied via one of the migrations so you can play with it by going to the Django Admin panel (see above how to create a superuser account) and the GraphiQL IDE.
FAQs
an automatic GraphQL API for all the models in a Django project
We found that django-auto-graphql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.