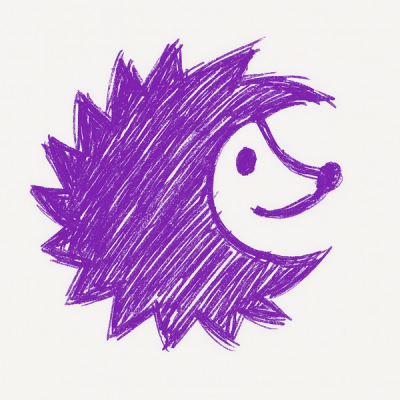
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
The simple sitemap generator for Python projects.
pip install dynamic-sitemap
from datetime import datetime
from dynamic_sitemap import SimpleSitemap, ChangeFreq
urls = [
'/',
{'loc': '/contacts', 'changefreq': ChangeFreq.NEVER.value},
{'loc': '/about', 'priority': 0.9, 'lastmod': datetime.now().isoformat()},
]
sitemap = SimpleSitemap('https://mysite.com', urls)
# or sitemap.render()
sitemap.write('static/sitemap.xml')
Only FlaskSitemap is implemented yet, so there is an example:
from dynamic_sitemap import FlaskSitemap
from flask import Flask
app = Flask(__name__)
sitemap = FlaskSitemap(app, 'https://mysite.com')
sitemap.build()
Then run your server and visit http://mysite.com/sitemap.xml.
The basic example with some Models:
from dynamic_sitemap import ChangeFreq, FlaskSitemap
from flask import Flask
from models import Post, Tag
app = Flask(__name__)
sitemap = FlaskSitemap(app, 'https://mysite.com', orm='sqlalchemy')
sitemap.config.TIMEZONE = 'Europe/Moscow'
sitemap.ignore('/edit', '/upload')
sitemap.add_items(
'/contacts',
{'loc': '/faq', 'changefreq': ChangeFreq.MONTHLY.value, 'priority': 0.4},
)
sitemap.add_rule('/blog', Post, loc_from='slug', priority=1.0)
sitemap.add_rule('/blog/tag', Tag, loc_from='id', changefreq='daily')
sitemap.build()
Also you can set configurations from your class (and it's preferred):
from dynamic_sitemap import ChangeFreq, FlaskSitemap
from flask import Flask
from models import Product
class Config:
FILENAME = 'static/sitemap.xml'
IGNORED = {'/admin', '/back-office', '/other-pages'}
ALTER_PRIORITY = 0.1
app = Flask(__name__)
sitemap = FlaskSitemap(app, 'https://myshop.org', config=Config)
sitemap.add_items(
'/contacts',
{'loc': '/about', 'changefreq': ChangeFreq.MONTHLY.value, 'priority': 0.4},
)
sitemap.add_rule('/goods', Product, loc_from='id', lastmod_from='updated')
sitemap.build()
Not supported yet:
/page/<int:user_id>/<str:slug>
Check out the Changelog.
Feel free to suggest any improvements :)
Fork this repository, clone it and install dependencies:
pip install -r requirements/all.txt
Checkout to a new branch, add your feature and some tests, then try:
make precommit
If the result is ok, create a pull request to "dev" branch of this repo with a detailed description.
Done!
FAQs
The sitemap generator for Python projects.
We found that dynamic-sitemap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.