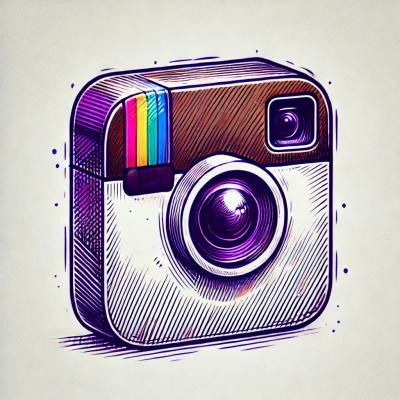
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
emval
is a blazingly fast email validator written in Rust with Python bindings, offering performance improvements of 100-1000x over traditional validators.
python-email-validator
, verify-email
, and pyIsEmail
.Install emval
from PyPI:
pip install emval
or use emval
in a Rust project:
cargo add emval
To validate an email address in Python:
from emval import validate_email, EmailValidator
email = "example@domain.com"
try:
# Check if the email is valid.
val_email = validate_email(email)
# Utilize the normalized form for storage.
normalized_email = val_email.normalized
except Exception as e:
# Example: "Invalid Local Part: Quoting the local part before the '@' sign is not permitted in this context."
print(str(e))
The same code in Rust:
use emval::{validate_email, ValidationError};
fn main() -> Result<(), ValidationError> {
let email = "example@domain.com";
let val_email = validate_email(email)?;
let normalized_email = val_email.normalized;
Ok(())
}
Customize email validation behavior using the EmailValidator
class:
from emval import EmailValidator
emval = EmailValidator(
allow_smtputf8=False,
allow_empty_local=True,
allow_quoted_local=True,
allow_domain_literal=True,
deliverable_address=False,
)
email = "user@[192.168.1.1]"
try:
validated_email = emval.validate_email(email)
print(validated_email)
except Exception as e:
print(str(e))
The same code in Rust:
use emval::{EmailValidator, ValidationError};
fn main() -> Result<(), ValidationError> {
let emval = EmailValidator {
allow_smtputf8: false,
allow_empty_local: true,
allow_quoted_local: true,
allow_domain_literal: true,
deliverable_address: false,
};
let email = "example@domain.com";
let validated_email = emval.validate_email(email)?;
Ok(())
}
allow_smtputf8
: Allows internationalized email addresses.allow_empty_local
: Allows an empty local part (e.g., @domain.com
).allow_quoted_local
: Allows quoted local parts (e.g., "user name"@domain.com
).allow_domain_literal
: Allows domain literals (e.g., [192.168.0.1]
).deliverable_address
: Checks if the email address is deliverable by verifying the domain's MX records.emval adheres to the syntax rules defined in RFC 5322 and RFC 6531. It supports both ASCII and internationalized characters.
emval converts non-ASCII domain names into their ASCII "Punycode" form according to IDNA 2008. This ensures compatibility with systems that do not support Unicode.
emval allows international characters in the local part of email addresses, following RFC 6531. It offers options to handle environments without SMTPUTF8 support.
emval rejects unsafe Unicode characters to enhance security, preventing display and interpretation issues.
emval normalizes email addresses to ensure consistency:
This project draws inspiration from python-email-validator. While python-email-validator
is more comprehensive, emval
aims to provide a faster solution.
For questions and issues, please open an issue in the GitHub issue tracker.
emval is licensed under the MIT License. See the LICENSE file for more details.
FAQs
emval is a blazingly fast email validator
We found that emval demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.