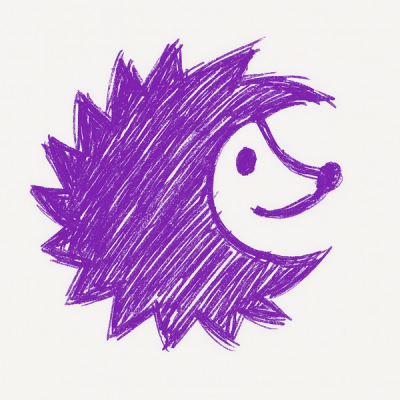
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Tools for encryption and decryption, signing and verification. Use symmetric and asymmetric (RSA-based) encryption.
Tools for encryption and decryption, signing and verification. Use symmetric and asymmetric (RSA-based) encryption.
pip install encryptiontools
from encryptiontools.encryption import AsymmetricEncrypter, AsymmetricDecrypter
from encryptiontools.utils import generate_key_pair
public_key, private_key = generate_key_pair(512)
data = {'message': 'hello asymmetric encryption'}
encrypter = AsymmetricEncrypter.create(public_key.save_pkcs1()) # or AsymmetricEncrypter(public_key)
decrypter = AsymmetricDecrypter.create(private_key.save_pkcs1()) # or AsymmetricDecrypter(private_key)
encrypted = encrypter.encrypt(data)
decrypted = decrypter.decrypt(encrypted)
assert decrypted['message'] == 'hello asymmetric encryption'
from encryptiontools.encryption import SymmetricEncrypter
key = b'0123456789abcdef'
data = {'message': 'hello symmetric encryption'}
encrypter = SymmetricEncrypter.create(key) # or SymmetricEncrypter(key)
encrypted = encrypter.encrypt(data)
decrypted = encrypter.decrypt(encrypted)
assert decrypted['message'] == 'hello symmetric encryption'
Asymmetric key pair is used to encrypt/decrypt internal (symmetric) key, internal key is used to decrypt data.
from encryptiontools.encryption import CombinedEncrypter, CombinedDecrypter
from encryptiontools.utils import generate_key_pair
public_key, private_key = generate_key_pair(512)
data = {'message': 'hello combined encryption'}
encrypter = CombinedEncrypter.create(public_key.save_pkcs1()) # or CombinedEncrypter(public_key)
decrypter = CombinedDecrypter.create(private_key.save_pkcs1()) # or CombinedDecrypter(private_key)
encrypted = encrypter.encrypt(data)
decrypted = decrypter.decrypt(encrypted)
assert decrypted['message'] == 'hello combined encryption'
from encryptiontools.signature import Signer, Verifier
from encryptiontools.utils import generate_key_pair
from encryptiontools.exceptions import VerificationError
public_key, private_key = generate_key_pair(512)
data = {'message': 'hello signing and verification'}
signer = Signer.create(private_key.save_pkcs1()) # or Signer(private_key)
verifier = Verifier.create(public_key.save_pkcs1()) # or Verifier(public_key)
signature = signer.sign(data)
try:
verifier.verify(data, signature)
assert True
except VerificationError:
assert False
FAQs
Tools for encryption and decryption, signing and verification. Use symmetric and asymmetric (RSA-based) encryption.
We found that encryptiontools demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.