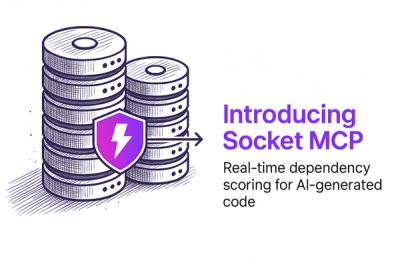
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Streamlined, no-fuss queue workers for Python.
Eventide is a streamlined, easy-to-setup framework for building queue workers. It lets you consume messages, schedule messages with cron, handle retries, and manage your worker lifecycle simply and reliably.
# Base installation
pip install eventide
# Choose a queue provider:
pip install eventide[sqs] # AWS SQS support
pip install eventide[cloudflare] # Cloudflare Queues support
# Optional features:
pip install eventide[cron] # Cron scheduling
pip install eventide[watch] # Dev autoreload
pip install eventide[core] # Installs all optional features (not queue providers)
A minimal Eventide app and handler:
from eventide import Eventide, EventideConfig, Message, SQSQueueConfig
app = Eventide(
config=EventideConfig(
queue=SQSQueueConfig(
region="us-east-1",
url="https://sqs.us-east-1.amazonaws.com/123456789012/my-queue",
buffer_size=10, # internal buffer queue
),
)
)
@app.handler("body.event == 'hello'")
def hello_world(message: Message) -> None:
print("Hello ", message.body.get("content", "World"))
Run:
eventide run -a app:app
# or using autoreload (recommended for development)
eventide run -a app:app --reload
Handlers process messages matching JMESPath expressions and can define retry behavior.
Example:
@app.handler(
"body.type == 'email' && body.event == 'send'",
retry_limit=5,
retry_for=[ValueError, TimeoutError],
retry_min_backoff=1.0,
retry_max_backoff=30.0,
)
def send_email(message):
print("Sending email:", message.body)
You can define functions decorated with @app.cron
to schedule the sending of messages.
Each cron function generates and returns the message body that Eventide will automatically enqueue to your configured queue provider at the scheduled time.
Example:
@app.cron("*/5 * * * * *") # every 5 seconds
def send_heartbeat():
return {"type": "heartbeat"}
Run the cron process separately from the worker process:
eventide cron -a app:app
# or using autoreload (recommended for development)
eventide cron -a app:app --reload
Workers and the cron scheduler are independent, allowing you to scale your workers without duplicating scheduled messages.
Hooks allow you to react to important lifecycle moments:
@app.on_start
def startup():
print("App is starting")
@app.on_shutdown
def shutdown():
print("App is shutting down")
@app.on_message_failure
def on_failure(message, exc):
print(f"Failed handling {message.id}: {exc}")
Available hooks:
on_start
on_shutdown
on_message_received
on_message_success
on_message_failure
Eventide currently has built-in support for:
More providers will be added over time.
You can also implement custom providers by extending the Queue
base class.
FAQs
Fast and simple queue workers
We found that eventide demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.