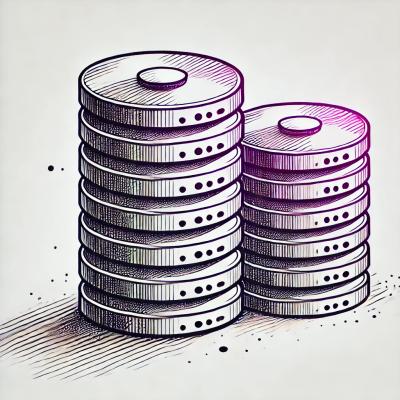
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
A high-performance, generic CRUD operations package combining SQLAlchemy async ORM with Redis caching. Features type-safe CRUD operations, automatic cache invalidation, pagination support, and Pydantic integration for schema validation. Ideal for FastAPI backends and read-heavy applications requiring optimized database access.
A high-performance, generic CRUD operations package combining SQLAlchemy async ORM with Redis caching. Features type-safe CRUD operations, automatic cache invalidation, pagination support, and Pydantic integration for schema validation. Ideal for FastAPI backends and read-heavy applications requiring optimized database access.
pip install fast-rdb
from fast_rdb import CRUDBase
from sqlalchemy.ext.asyncio import AsyncSession
from redis.asyncio import Redis
from pydantic import BaseModel
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.exc import IntegrityError, NoResultFound
Base = declarative_base()
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
class UserCreate(BaseModel):
name: str
email: str
class UserUpdate(BaseModel):
name: str | None = None
email: str | None = None
class UserResponse(BaseModel):
id: int
name: str
email: str
class UserCRUD(CRUDBase[User, UserCreate, UserUpdate, UserResponse]):
def __init__(self):
super().__init__(
model=User,
response_schema=UserResponse,
pattern="user:{id}",
list_pattern="users:page:{page}:limit:{limit}",
exp=3600,
invalidate_pattern_prefix="user:*"
)
# Usage
async def main():
db = AsyncSession(...) # Your SQLAlchemy async session
redis = Redis(...) # Your Redis client
user_crud = UserCRUD()
# Create a user
try:
new_user = await user_crud.create(
db=db,
redis=redis,
obj_in=UserCreate(name="John Doe", email="john@example.com")
)
except IntegrityError:
raise DuplicateValueException(detail="User already exists")
# Get a user
user = await user_crud.get(db=db, redis=redis, id=1)
if not user:
raise NotFoundException(detail="User not found")
# Update a user
try:
updated_user = await user_crud.update(
db=db,
redis=redis,
obj_in=UserUpdate(name="Jane Doe"),
id=1
)
except NoResultFound:
raise NotFoundException(detail="User not found")
# Delete a user
try:
await user_crud.delete(db=db, redis=redis, id=1)
except NoResultFound:
raise NotFoundException(detail="User not found")
# Get paginated users
users = await user_crud.get_multi(
db=db,
redis=redis,
limit=10,
page=1,
order_by="name"
)
return user_crud.paginate(data=users, limit=10, page=1)
The main class providing CRUD operations with Redis caching.
model
(Type[ModelType]): SQLAlchemy model class that represents your database tableresponse_schema
(Type[ResponseSchemaType]): Pydantic model for response serializationpattern
(str): Redis key pattern for single record caching (e.g., "user:{id}")list_pattern
(str): Redis key pattern for list caching (e.g., "users:page:{page}:limit:{limit}")exp
(int): Redis key expiration time in secondsinvalidate_pattern_prefix
(str): Pattern prefix for cache invalidation (e.g., "user:*")async def create(
db: AsyncSession,
redis: Redis,
obj_in: CreateSchemaType,
**kwargs: Any
) -> ResponseSchemaType
Creates a new record in the database and caches it in Redis.
db
: SQLAlchemy async sessionredis
: Redis client instanceobj_in
: Pydantic model with data to create**kwargs
: Additional filters for the recordasync def get(
db: AsyncSession,
redis: Redis,
**kwargs: Any
) -> ResponseSchemaType | None
Retrieves a single record from cache or database.
db
: SQLAlchemy async sessionredis
: Redis client instance**kwargs
: Filters to identify the recordasync def get_multi(
db: AsyncSession,
redis: Redis,
limit: int = 10,
page: int = 1,
order_by: Optional[str] = None,
ascending: bool = True,
**kwargs: Any
) -> List[ResponseSchemaType]
Retrieves multiple records with pagination and optional ordering.
db
: SQLAlchemy async sessionredis
: Redis client instancelimit
: Number of records per pagepage
: Page numberorder_by
: Field to order byascending
: Sort order**kwargs
: Additional filtersasync def update(
db: AsyncSession,
redis: Redis,
obj_in: UpdateSchemaType,
**matches: Any
) -> ResponseSchemaType
Updates an existing record.
db
: SQLAlchemy async sessionredis
: Redis client instanceobj_in
: Pydantic model with update data**matches
: Filters to identify the recordasync def delete(
db: AsyncSession,
redis: Redis,
**kwargs: Any
) -> None
Deletes a record from database and cache.
db
: SQLAlchemy async sessionredis
: Redis client instance**kwargs
: Filters to identify the recordasync def create_multi(
db: AsyncSession,
instances: list[CreateSchemaType]
) -> Sequence[ModelType]
Creates multiple records in a single transaction.
db
: SQLAlchemy async sessioninstances
: List of Pydantic models to create@staticmethod
def paginate(
data: List[Any],
limit: int,
page: int
) -> PaginatedResponse[Any]
Helper method to create paginated responses.
data
: List of items to paginatelimit
: Items per pagepage
: Current page numberThe package integrates with common database exceptions:
IntegrityError
: Raised when trying to create a duplicate recordNoResultFound
: Raised when a record is not found during update or delete operationsExample error handling:
try:
result = await user_crud.get(db=db, redis=redis, id=1)
if not result:
raise NotFoundException(detail="User not found")
return result
except NoResultFound:
raise NotFoundException(detail="User not found")
This project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! Please feel free to submit a Pull Request.
FAQs
A high-performance, generic CRUD operations package combining SQLAlchemy async ORM with Redis caching. Features type-safe CRUD operations, automatic cache invalidation, pagination support, and Pydantic integration for schema validation. Ideal for FastAPI backends and read-heavy applications requiring optimized database access.
We found that fast-rdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.