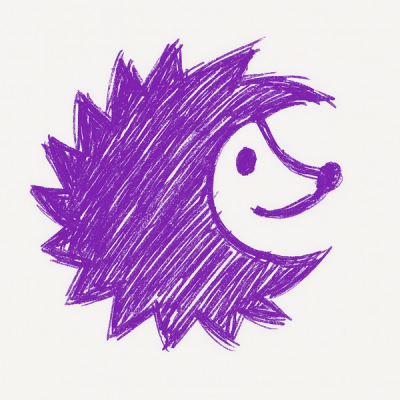
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
A package to quickly hot reload large Python projects. Currently for Linux & OSX.
Once your project gets to a certain size, the main overhead of application startup is typically the loading of 3rd party packages. Packages can do varying degrees of global initialization when they're imported. They can also require 100s or 1000s of additional files, which the AST parser has to step through one by one. Cached bytecode can help but it's not a silver bullet.
Eventually your bootup can take 5-10s just to load these modules. Fine for a production service that's intended to run continuously, but horrible for a development experience when you have to reboot after changes. Firehot solves this by importing dependencies once, then forking your environment for each exec. Think of it like building a docker image then executing it whenever you make a change.
from firehot import isolate_imports
from os import getpid, getppid
def run_under_environment(value: int):
parent_pid = getppid()
print(f"Running with value: {value} (pid: {getpid()}, parent_pid: {parent_pid})")
with isolate_imports("my_package") as environment:
# Each exec will be run in a new process with the environment isolated, inheriting
# the package's third party imports without having to re-import them from scratch.
context1 = environment.exec(run_under_environment, 1)
context2 = environment.exec(run_under_environment, 2)
# These can potentially be long running - to wait on the completion status, you can do:
result1 = environment.communicate_isolated(context1)
result2 = environment.communicate_isolated(context2)
# If you change the underlying file on disk to add an import, you can run update_environment.
# If the files on disk don't add any new imports, it will be a no-op and keep the current
# environment process running.
environment.update_environment()
# Subsequent execs will use the updated environment.
context3 = environment.exec(run_under_environment, 3)
result3 = environment.communicate_isolated(context3)
By default, Firehot logs at the warn
level, but you can adjust this by setting the FIREHOT_LOG_LEVEL
environment variable. Most of these logs come from the Rust code.
Available log levels (from most to least verbose):
trace
: Extremely detailed information, useful for debugging specific issuesdebug
: Detailed information useful during developmentinfo
: General information about what's happening (default)warn
: Warning messages for potential issueserror
: Error messages for actual problemsExample usage:
# Set to debug level for more detailed logs
FIREHOT_LOG_LEVEL=debug python your_script.py
# Set to error level for minimal logs
FIREHOT_LOG_LEVEL=error python your_script.py
You launch Firehot by pointing it to your package name, which we resolve internally to a disk path that contains your code. From there, our Rust logic takes over. The pipeline will parse this directory recursively for all Python files, then parse the code's AST to determine which imports are used by your project.
It will then launch a continuously running process that caches only the 3rd party packages/modules. We think of this as the "template" process because it establishes the environment that will be used to run your code. None of your user code is run in this process.
When code changes are made in your project, we will:
To test how firehot works with a real project, we bundle a demopackage
and external-package
library in this repo.
# To do a regular, fast development build
(make build-develop && cd demopackage && uv run test-hotreload)
# To pass the args we use in a release
(make build-develop MATURIN_ARGS="--release --strip" && cd demopackage && uv run test-hotreload)
cargo test
FAQs
Wicked fast hot reloading
We found that firehot demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.