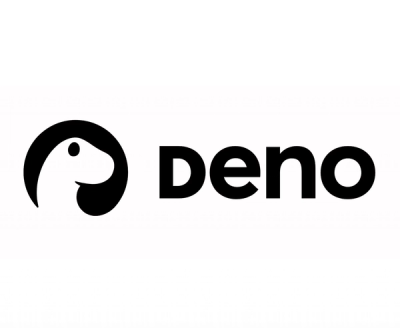
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
A Python library for static arrays with dynamic memory allocation like malloc, realloc, and free, and various array operations like searching, sorting, and mathematics.
FlexArray
is a Python library that provides an array-like structure with dynamic memory allocation features similar to C's malloc
, realloc
, and free
.
You can install the package via pip:
pip install flexarray
Implementing Array with flexarray
from flexarray.array import Array
# Create an array with initial size 3
arr = Array(3)
arr[0] = 10
arr[1] = 20
print(arr) # Array([10, 20, None])
# Automatic dynamic resizing
arr[5] = 50
print(arr) # Array([10, 20, None, None, None, 50])
# Malloc: Allocate memory dynamically
arr.malloc(5)
print(arr) # Array([None, None, None, None, None])
# Realloc: Resize the array
arr.realloc(10)
print(arr) # Array([None, None, None, None, None, None, None, None, None, None])
# Free: Deallocate memory
arr.free()
print(arr) # Array([])
Using Mathematics and Sorting on FlexArray
from flexarray.array import Array
from flexarray.mathematics import (
SumOfElements, AverageOfElements, MaxElement, MinElement,
ProductOfElements, MedianOfElements, ModeOfElements
)
from flexarray.sorting import BubbleSort, SelectionSort, InsertionSort
# Step 1: Create a FlexArray with initial elements
arr = Array(6)
arr[0] = 10
arr[1] = 20
arr[2] = 30
arr[3] = 40
arr[4] = 50
arr[5] = 60
# Step 2: Perform mathematical operations
print("Sum of elements:", SumOfElements(arr)) # Output: 210
print("Average of elements:", AverageOfElements(arr)) # Output: 35.0
print("Maximum element:", MaxElement(arr)) # Output: 60
print("Minimum element:", MinElement(arr)) # Output: 10
print("Product of elements:", ProductOfElements(arr)) # Output: 720000000
print("Median of elements:", MedianOfElements(arr)) # Output: 35.0
print("Mode of elements:", ModeOfElements(arr)) # Output: None (since no repeating elements)
# Step 3: Sorting FlexArray using different algorithms
# Bubble Sort
BubbleSort(arr)
print("Array after Bubble Sort:", arr)
# Selection Sort
arr.realloc(6) # Reset array for demonstration
arr[0], arr[1], arr[2], arr[3], arr[4], arr[5] = 50, 40, 30, 20, 10, 60 # Unsorted
SelectionSort(arr)
print("Array after Selection Sort:", arr)
# Insertion Sort
arr.realloc(6) # Reset array again
arr[0], arr[1], arr[2], arr[3], arr[4], arr[5] = 60, 50, 40, 30, 20, 10 # Unsorted
InsertionSort(arr)
print("Array after Insertion Sort:", arr)
FAQs
A Python library for static arrays with dynamic memory allocation like malloc, realloc, and free, and various array operations like searching, sorting, and mathematics.
We found that flexarray demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.