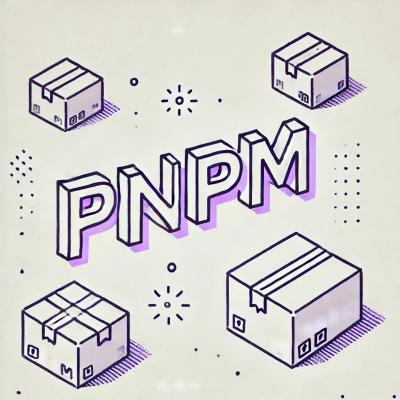
Security News
pnpm 10.12 Introduces Global Virtual Store and Expanded Version Catalogs
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
gradient-agreement-filtering
Advanced tools
This package implements the Gradient Agreement Filtering (GAF) optimization algorithm.
GAF is a novel optimization algorithm that improves gradient-based optimization by filtering out gradients of data batches that do not agree with each other and nearly eliminates the need for a validation set without risk of overfitting (even with noisy labels). It bolts on top of existing optimization procedures such as SGD, SGD with Nesterov momentum, Adam, AdamW, RMSProp, etc and outperforms in all cases. Full paper here:
TODO: Insert arxiv paper link.
You can install via:
git clone https://github.com/<insert your username>/gradient_agreement_filtering.git
cd gradient_agreement_filtering
pip install .
or via pip:
pip install gradient-agreement-filtering
We provide two ways to easily incorporate GAF into your existing training.
step_GAF(): If you want to use GAF inside your existing train loop, you can just replace your typical:
...
optimizer.zero_grad()
outputs = model(batch)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
...
with one call to step_GAF() as per below:
from gradient_agreement_filtering import step_GAF
...
results = step_GAF(model,
optimizer,
criterion,
list_of_microbatches,
wandb=True,
verbose=True,
cos_distance_thresh=0.97,
device=gpu_device)
...
train_GAF():
If you want to use GAF as the train loop, you can just replace your typical hugging face / keras style interface:
trainer.Train()
with one call to train_GAF() as per below:
from gradient_agreement_filtering import train_GAF
...
train_GAF(model,
args,
train_dataset,
val_dataset,
optimizer,
criterion,
wandb=True,
verbose=True,
cos_distance_thresh=0.97,
device=gpu_device)
...
For all of the scripts below, if you want to run with wandb, you can either fill in the:
os.environ["WANDB_API_KEY"] = "<your-wandb-api-key>"
Or you can prepend any of the calls below with:
WANDB_API_KEY=<your-wandb-api-key> python *.py
Or you can login on the system first then run the .py via:
wandb login <your-wandb-api-key>
Or you can run without it. Choice is yours.
Now please review the examples below.
This file uses step_GAF() to train a ResNet18 model on the CIFAR-100 dataset using PyTorch with the ability to add noise to the labels to observe how GAF performs under noisy conditions. The code supports various optimizers and configurations, allowing you to experiment with different settings to understand the impact of GAF on model training.
Example call:
python examples/1_cifar_100_train_loop_exposed.py --GAF True --optimizer "SGD+Nesterov+val_plateau" --learning_rate 0.01 --momentum 0.9 --nesterov True --wandb True --verbose True --num_samples_per_class_per_batch 1 --num_batches_to_force_agreement 2 --label_error_percentage 0.15 --cos_distance_thresh 0.97
This file uses train_GAF() to train a ResNet18 model on the CIFAR-100 dataset using PyTorch just to show how it works.
Example call:
python examples/2_cifar_100_trainer.py
This file uses step_GAF() to train a ResNet34 model on the CIFAR-100N-Fine dataset using PyTorch to observe how GAF performs under typical labeling noise. The code supports various optimizers and configurations, allowing you to experiment with different settings to understand the impact of GAF on model training.
Example call:
python examples/3_cifar_100N_Fine_train_loop_exposed.py --GAF True --optimizer "SGD+Nesterov+val_plateau" --cifarn True --learning_rate 0.01 --momentum 0.9 --nesterov True --wandb True --verbose True --num_samples_per_class_per_batch 2 --num_batches_to_force_agreement 2 --cos_distance_thresh 0.97
To cite this work, please use the following BibTeX entry:
TODO
Insert bibtex
This package is licensed under the MIT license. See LICENSE for details.
FAQs
Gradient Agreement Filtering (GAF) Package
We found that gradient-agreement-filtering demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.