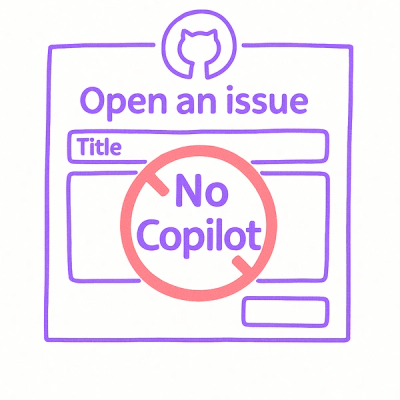
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
GridPath is a Python library for calculating grid-based paths and intersection points between two coordinates. It's particularly useful for applications requiring precise path tracking on a grid system, such as robotics, game development, or computer graphics.
GridPath is a Python library for calculating grid-based paths and intersection points between two coordinates. It's particularly useful for applications requiring precise path tracking on a grid system, such as robotics, game development, or computer graphics.
pip install gridpath2
Basic usage example:
from gridpath2 import grid
# Calculate path between two points
result = grid(x1=1.5, y1=2.3, x2=4.7, y2=6.8)
# Access the results
grid_points = result["grid"] # List of grid points traversed
intersections = result["intersect"] # List of exact intersection points
The function returns a dictionary containing:
grid
: List of grid points the path traverses throughintersect
: List of exact coordinates where the path intersects with grid linespath = grid(1.5, 2.0, 4.5, 2.0)
# Returns path along y=2
path = grid(2.0, 1.5, 2.0, 4.5)
# Returns path along x=2
path = grid(1.0, 1.0, 3.0, 3.0)
# Returns diagonal path with slope=1
path = grid(1.5, 2.3, 4.7, 6.8)
# Returns optimal grid path with precise intersections
def grid(x1: float, y1: float, x2: float, y2: float) -> dict:
"""Calculate grid path and intersection points between two coordinates.
Args:
x1, y1: Starting point coordinates
x2, y2: Ending point coordinates
Returns:
dict: Dictionary containing:
- "grid": List of grid points [[x1, y1], [x2, y2], ...]
- "intersect": List of intersection points [[x1, y1], [x2, y2], ...]
"""
The library handles several special cases:
For general cases, the algorithm:
MIT License
Contributions are welcome! Please feel free to submit a Pull Request.
FAQs
GridPath is a Python library for calculating grid-based paths and intersection points between two coordinates. It's particularly useful for applications requiring precise path tracking on a grid system, such as robotics, game development, or computer graphics.
We found that gridpath2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.