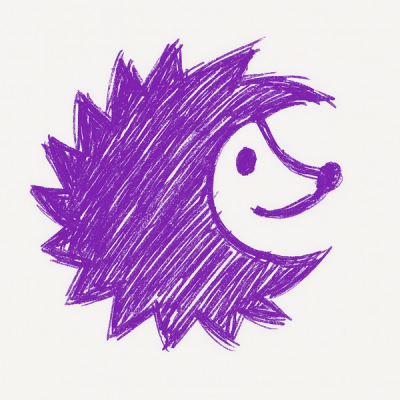
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
The python functional programming library you need
pip install haskellian
Iter[A]
from haskellian import Iter, iter as I
@I.lift
def gen():
for i in range(100000000000):
yield i
gen() \
.map(lambda x: 2*x) \
.filter(lambda x: x % 2 == 0) \
.batch(2) \
.skip(1)
#.sync() # don't recommend it...
# Iter([(4, 6), (8, 10), (12, 14), (16, 18), (20, 22), ...])
Either[L, R]
from haskellian import Either, either as E
def fetch_users() -> Either[str, list[str]]:
...
def fetch_user(id: str) -> Either[str, dict]:
...
def parse_user(user: dict) -> Either[str, User]:
...
@E.do()
def print_one():
users = fetch_users().unsafe()
raw_user = fetch_user(users[0]).unsafe()
user = parse_user(raw_user).unsafe()
print(user)
Explanation:
.unsafe()
raises unwraps the value or raises an IsLeft
exception@E.do()
wraps the function in a try...except IsLeft
block, returning the possibly raised Left
Dict[A]
from haskellian import Dict
Dict({ 'a': 1, 'b': 2, 'c': 4 }) \
.filter_v(lambda v: v % 2 == 0) \
.evolve({ 'c': lambda x: -x })
.map_v(lambda v: 2*v)
# Dict({ 'b': 4, 'c': -8 })
AsynIter[A]
from haskellian import AsynIter, asyn_iter as AI
@AI.lift
async def gen():
for i in range(10):
yield i
await gen() \
.map(lambda x: 2*x) \
.filter(lambda x: x % 2 == 0) \
.batch(2) \
.skip(1) \
.sync()
FAQs
The functional programming library you need
We found that haskellian demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.