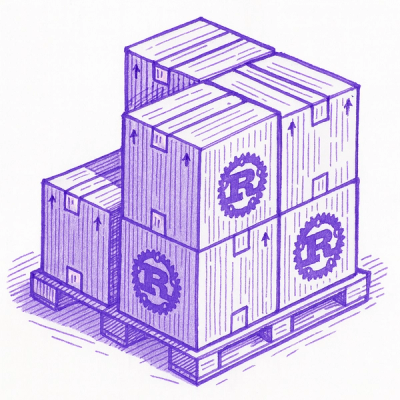
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
The hgvs package provides a Python library to parse, format, validate, normalize, and map sequence variants according to Variation Nomenclature (aka Human Genome Variation Society) recommendations.
Specifically, the hgvs package focuses on the subset of the HGVS recommendations that precisely describe sequence-level variation relevant to the application of high-throughput sequencing to clinical diagnostics. The package does not attempt to cover the full scope of HGVS recommendations. Please refer to issues for limitations.
Wang M, Callenberg KM, Dalgleish R, Fedtsov A, Fox N, Freeman PJ, et al.
hgvs: A Python package for manipulating sequence variants using HGVS nomenclature: 2018 Update.
Hum Mutat. 2018. doi:10.1002/humu.23615
hgvs>=1.5,<1.6
. hgvs uses Semantic
Versioning.Important: For more detailed installation and configuration instructions, see the HGVS readthedocs
libpq
python3
postgresql
Examples for installation:
MacOS :
brew install libpq
brew install python3
brew install postgresql@14
Ubuntu :
sudo apt install gcc libpq-dev python3-dev
By default, hgvs uses remote data sources, which makes installation easy. If you would like to use local instances of the data sources, see the readthedocs.
Create a virtual environment using your preferred method.
Example:
python3 -m venv venv
Run the following commands in your virtual environment:
source venv/bin/activate
pip install --upgrade setuptools
pip install hgvs
See Installation instructions for details, including instructions for installing Universal Transcript Archive (UTA) and SeqRepo locally.
See examples and readthedocs for usage.
The hgvs package is a community effort. Please see Contributing to get started in submitting source code, tests, or documentation. Thanks for getting involved!
Existing tests use a cache that is committed with the repo to ensure that tests do not require external networking. To develop new tests, which requires loading the cache, you should install UTA and Seqrepo (and the rest service) locally.
docker compose --project-name biocommons -f $PWD/misc/docker-compose.yml up
IMPORTANT: Loading the test caches is currently hampered b
#551,
#760, and
#761. To load reliably, use
make test-relearn-iteratively
for now.
Other packages that manipulate HGVS variants:
FAQs
HGVS Parser, Formatter, Mapper, Validator
We found that hgvs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.