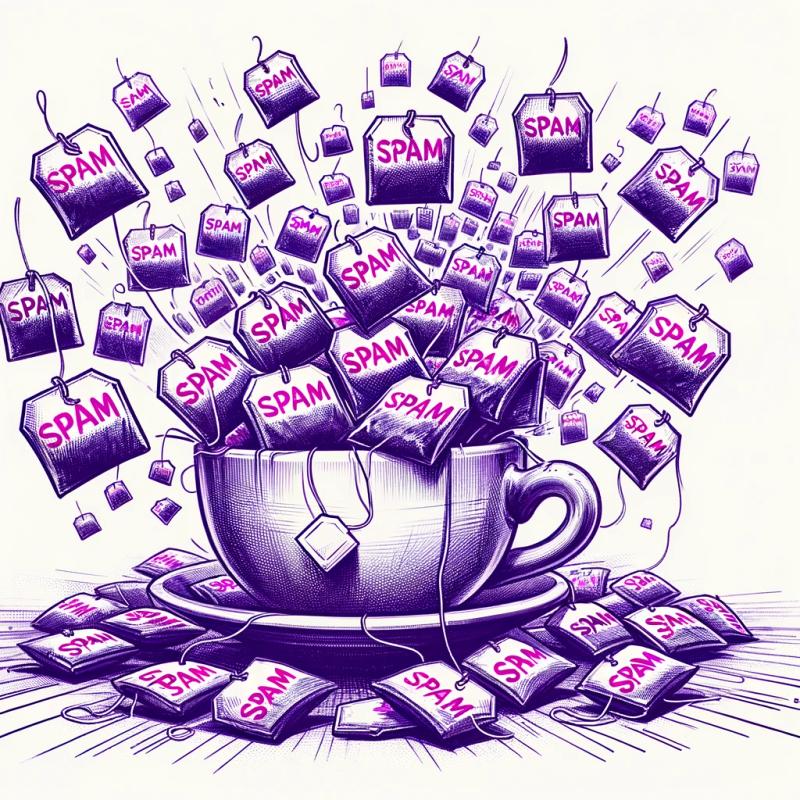
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
infobip-api-python-sdk
Readme
Client SDK to use the Infobip API with Python.
This package enables you to use multiple Infobip communication channels, like SMS, MMS, WhatsApp, Email, etc.
The following communication channels are supported:
The following platform management APIs are supported:
More APIs to be added in the near future.
Currently, infobip-api-python-sdk only supports API Key authentication, and the key needs to be passed during client creation. This will most likely change with future versions, once more authentication methods are included.
To install infobip SDK you will need to run:
pip install infobip-api-python-sdk
Details of the package can be found in the PyPI page.
To use the package you'll need an Infobip account. If you don't already have one, you can create a free trial account here.
In this example, we will show how to send a WhatsApp text message. Other channels can be used in a similar way. The first step is to import the necessary channel, in this case WhatsApp channel.
from infobip_channels.whatsapp.channel import WhatsAppChannel
Now you can create instance of WhatsAppChannel
with your base_url
and api_key
.
c = WhatsAppChannel.from_auth_params({
"base_url": "<your_base_url>",
"api_key": "<your_api_key>"
})
Alternatively, you can create the instance from the environment, having the IB_BASE_URL
and IB_API_KEY
variables
set, like this:
c = WhatsAppChannel.from_env()
After that you can access all the methods from WhatsAppChannel
.
To send text message you can use send_text_message
method and add correct payload:
response = c.send_text_message(
{
"from": "<WhatsApp sender number from your Infobib account>",
"to": "<Number that will receive WhatsApp message>",
"messageId": "a28dd97c-1ffb-4fcf-99f1-0b557ed381da",
"content": {
"text": "Some text"
},
"callbackData": "Callback data",
"notifyUrl": "https://www.example.com/whatsapp"
}
)
We are adding samples in the samples folder, which you can use as a reference on how to use the SDK with real payloads.
For infobip-api-python-sdk
versioning we use
Semantic Versioning scheme.
Python 3.6 is the minimum supported version by this library.
Check out our contributing guide and code of conduct.
This library is distributed under the MIT license found in the License.
FAQs
Python sdk for Infobip's API
We found that infobip-api-python-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.