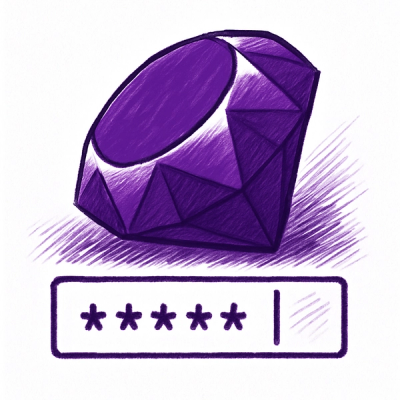
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
A Python library for interacting with the official Instagram Graph API. This client provides easy-to-use methods for authentication, user management, conversations, and messaging functionality.
Official instagram documentation
You can visit robosell to test how instagram integration works and features have
pip install instagram-client
Before using this library, you need to:
from instagram_client import InstagramClient
# Initialize the client
client = InstagramClient(
client_id="your_client_id",
client_secret="your_client_secret",
redirect_uri="your_redirect_uri",
scopes=["instagram_business_basic", "instagram_business_manage_messages"]
)
# For more detailed information visit https://developers.facebook.com/docs/instagram-platform/instagram-api-with-instagram-login
# Get the authorization URL
auth_url = client.get_auth_url()
# Also you can pass custom data to oauth url:
# auth_url = client.get_auth_url(state="user_{user_id}")
# Redirect user to auth_url for authorization
# After user authorization, exchange the code for an access token
login_response = client.retrieve_access_token(code="authorization_code")
# Exchange for a long-lived token
long_lived_token = client.exchange_long_lived_token() # long lived token expires in 60 days and short token expires in 30min
# Get authenticated user's profile
user_profile = client.get_me()
# Create new endpoint to redirect oauth url
# 1. Generate authentication URL
auth_url = client.get_auth_url(
enable_fb_login=1,
force_authentication=0,
state="optional_state_parameter"
)
# 2. After user authorization, exchange the code for an access token
login_response = client.retrieve_access_token(code="authorization_code")
print(f"Access Token: {login_response.access_token}")
# 3. Exchange for a long-lived token
long_lived_token = client.exchange_long_lived_token()
print(f"Long-lived Token: {long_lived_token.access_token}")
print(f"Token Expires in: {long_lived_token.expires_in} seconds")
# Get authenticated user's profile
me = client.get_me()
print(f"Username: {me.username}")
print(f"Followers: {me.followers_count}")
print(f"Following: {me.follows_count}")
# Get another user's profile
user_profile = client.get_profile_info(user_id="target_user_id")
print(f"Name: {user_profile.name}")
print(f"Is Following Business: {user_profile.is_user_follow_business}")
# Get all conversations
conversations = client.get_conversations()
for conversation in conversations:
print(f"Conversation ID: {conversation.id}")
print(f"Participants: {conversation.participants}")
# Get conversation with specific user
user_conversations = client.get_user_conversation(
user_id="target_user_id")
# Get messages from a conversation
messages = client.get_conversation_messages(
conversation_id="conversation_id",
desired_limit=100 # Optional: limit number of messages
)
for message in messages:
print(f"From: {message.from_}")
print(f"Message: {message.message}")
print(f"Time: {message.created_time}")
from instagram_client.schemes.conversation_schemes import SendMessageScheme
# Create message payload
message = SendMessageScheme(
message="Hello from Instagram API Client!",
recipient_id="target_user_id"
)
# Send message
response = client.send_message(
instagram_id="target_user_id",
payload=message
)
The library includes built-in error handling for common API issues:
from instagram_client.exceptions.http_exceptions import ForbiddenApiException
try:
token = client.retrieve_access_token(code="invalid_code")
except ForbiddenApiException as e:
print(f"Authentication failed: {e}")
For detailed information about the Instagram Graph API endpoints and features, visit the official Instagram Graph API documentation.
Contributions are welcome! Please feel free to submit a Pull Request.
Please note that this library handles sensitive authentication tokens. Always follow security best practices:
If you encounter any issues or have questions, please file an issue on the GitHub repository.
Email - erkinovabdulvoris101@gmail.com
Telegram - @abdulvoris_101
FAQs
Instagram official api 2.0 client by robosell.uz
We found that instagram-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.