kornia-rs: low level computer vision library in Rust

The kornia-rs
crate is a low level library for Computer Vision written in Rust 🦀
Use the library to perform image I/O, visualisation and other low level operations in your machine learning and data-science projects in a thread-safe and efficient way.
Getting Started
cargo run --bin hello_world -- --image-path path/to/image.jpg
use kornia::image::Image;
use kornia::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let image: Image<u8, 3> = F::read_image_any("tests/data/dog.jpeg")?;
println!("Hello, world! 🦀");
println!("Loaded Image size: {:?}", image.size());
println!("\nGoodbyte!");
Ok(())
}
Hello, world! 🦀
Loaded Image size: ImageSize { width: 258, height: 195 }
Goodbyte!
Features
- 🦀The library is primarly written in Rust.
- 🚀 Multi-threaded and efficient image I/O, image processing and advanced computer vision operators.
- 🔢 The n-dimensional backend is based on the
ndarray
crate. - 🐍 Python bindings are created with PyO3/Maturin.
- 📦 We package with support for Linux [amd64/arm64], Macos and WIndows.
- Supported Python versions are 3.7/3.8/3.9/3.10/3.11
Supported image formats
- Read images from AVIF, BMP, DDS, Farbeld, GIF, HDR, ICO, JPEG (libjpeg-turbo), OpenEXR, PNG, PNM, TGA, TIFF, WebP.
Image processing
- Convert images to grayscale, resize, crop, rotate, flip, pad, normalize, denormalize, and other image processing operations.
Video processing
- Capture video frames from a camera.
🛠️ Installation
>_ System dependencies
Dependeing on the features you want to use, you might need to install the following dependencies in your system:
turbojpeg
sudo apt-get install nasm
gstreamer
sudo apt-get install libgstreamer1.0-dev libgstreamer-plugins-base1.0-dev
** Check the gstreamr installation guide: https://docs.rs/gstreamer/latest/gstreamer/#installation
🦀 Rust
Add the following to your Cargo.toml
:
[dependencies]
kornia = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
Alternatively, you can use each sub-crate separately:
[dependencies]
kornia-core = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-io = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-image = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-imgproc = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
🐍 Python
pip install kornia-rs
Examples: Image processing
The following example shows how to read an image, convert it to grayscale and resize it. The image is then logged to a rerun
recording stream.
Checkout all the examples in the examples
directory to see more use cases.
use kornia::{image::{Image, ImageSize}, imgproc};
use kornia::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let image: Image<u8, 3> = F::read_image_any("tests/data/dog.jpeg")?;
let image_viz = image.clone();
let image_f32: Image<f32, 3> = image.cast_and_scale::<f32>(1.0 / 255.0)?;
let mut gray = Image::<f32, 1>::from_size_val(image_f32.size(), 0.0)?;
imgproc::color::gray_from_rgb(&image_f32, &mut gray)?;
let new_size = ImageSize {
width: 128,
height: 128,
};
let mut gray_resized = Image::<f32, 1>::from_size_val(new_size, 0.0)?;
imgproc::resize::resize_native(
&gray, &mut gray_resized,
imgproc::resize::InterpolationMode::Bilinear,
)?;
println!("gray_resize: {:?}", gray_resized.size());
let rec = rerun::RecordingStreamBuilder::new("Kornia App").connect()?;
let _ = rec.log("image", &rerun::Image::try_from(image_viz.data)?);
let _ = rec.log("gray", &rerun::Image::try_from(gray.data)?);
let _ = rec.log("gray_resize", &rerun::Image::try_from(gray_resized.data)?);
Ok(())
}
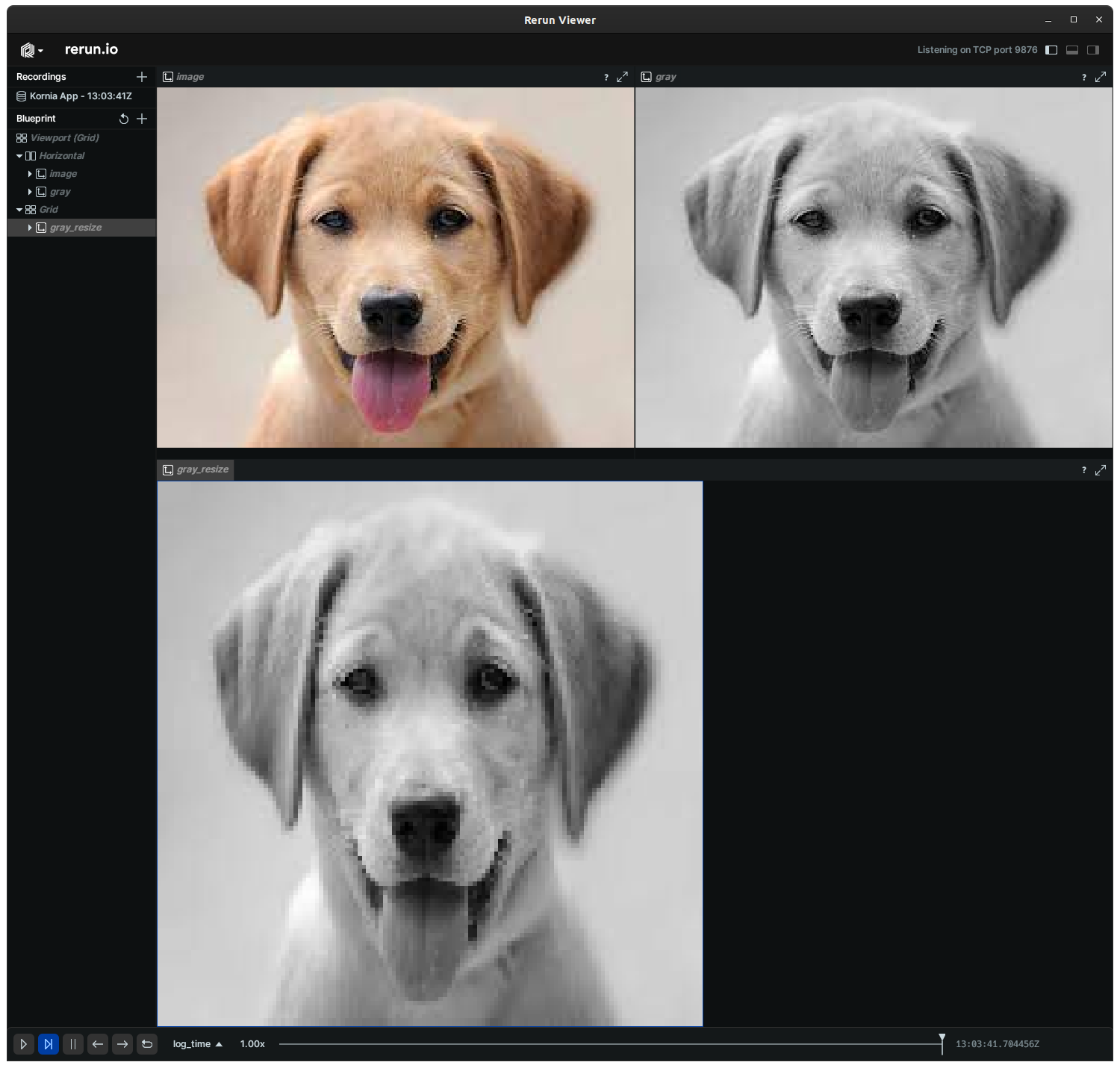
Python usage
Load an image, that is converted directly to a numpy array to ease the integration with other libraries.
import kornia_rs as K
import numpy as np
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
assert img.shape == (195, 258, 3)
img_t = torch.from_dlpack(img)
assert img_t.shape == (195, 258, 3)
Write an image to disk
import kornia_rs as K
import numpy as np
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
K.write_image_jpeg("dog_copy.jpeg", img)
Encode or decode image streams using the turbojpeg
backend
import kornia_rs as K
img = K.read_image_jpeg("dog.jpeg")
image_encoder = K.ImageEncoder()
image_encoder.set_quality(95)
img_encoded: list[int] = image_encoder.encode(img)
image_decoder = K.ImageDecoder()
decoded_img: np.ndarray = image_decoder.decode(bytes(image_encoded))
Resize an image using the kornia-rs
backend with SIMD acceleration
import kornia_rs as K
img = K.read_image_jpeg("dog.jpeg")
resized_img = K.resize(img, (128, 128), interpolation="bilinear")
assert resized_img.shape == (128, 128, 3)
🧑💻 Development
Pre-requisites: install rust
and python3
in your system.
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Clone the repository in your local directory
git clone https://github.com/kornia/kornia-rs.git
🦀 Rust
Compile the project and run the tests
cargo test
For specific tests, you can run the following command:
cargo test image
🐍 Python
To build the Python wheels, we use the maturin
package. Use the following command to build the wheels:
make build-python
To run the tests, use the following command:
make test-python
💜 Contributing
This is a child project of Kornia. Join the community to get in touch with us, or just sponsor the project: https://opencollective.com/kornia