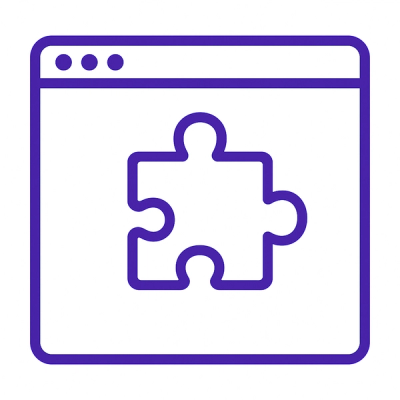
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
langgraph-checkpoint-aws
Advanced tools
A LangChain checkpointer implementation that uses Bedrock Session Management Service to enable stateful and resumable LangGraph agents.
A custom LangChain checkpointer implementation that uses Bedrock Session Management Service to enable stateful and resumable LangGraph agents through efficient state persistence and retrieval.
This package provides a custom checkpointing solution for LangGraph agents using AWS Bedrock Session Management Service. It enables:
You can install the package using pip:
pip install langgraph-checkpoint-aws
Or with Poetry:
poetry add langgraph-checkpoint-aws
Python >=3.9
langgraph-checkpoint >=2.0.0
langgraph >=0.2.55
boto3 >=1.37.3
from langgraph.graph import StateGraph
from langgraph_checkpoint_aws.saver import BedrockSessionSaver
# Initialize the saver
session_saver = BedrockSessionSaver(
region_name="us-west-2", # Your AWS region
credentials_profile_name="default", # Optional: AWS credentials profile
)
# Create a session
session_id = session_saver.session_client.create_session().session_id
# Use with LangGraph
builder = StateGraph(int)
builder.add_node("add_one", lambda x: x + 1)
builder.set_entry_point("add_one")
builder.set_finish_point("add_one")
graph = builder.compile(checkpointer=session_saver)
config = {"configurable": {"thread_id": session_id}}
graph.invoke(1, config)
BedrockSessionSaver
accepts the following parameters:
def __init__(
region_name: Optional[str] = None,
credentials_profile_name: Optional[str] = None,
aws_access_key_id: Optional[SecretStr] = None,
aws_secret_access_key: Optional[SecretStr] = None,
aws_session_token: Optional[SecretStr] = None,
endpoint_url: Optional[str] = None,
config: Optional[Config] = None,
)
region_name
: AWS region where Bedrock is availablecredentials_profile_name
: Name of AWS credentials profile to useaws_access_key_id
: AWS access key ID for authenticationaws_secret_access_key
: AWS secret access key for authenticationaws_session_token
: AWS session token for temporary credentialsendpoint_url
: Custom endpoint URL for the Bedrock serviceconfig
: Botocore configuration objectSetting Up Development Environment
git clone <repository-url>
cd libs/aws/langgraph-checkpoint-aws
make install_all
make install_dev # Basic development tools
make install_test # Testing tools
make install_lint # Linting tools
make install_typing # Type checking tools
make install_codespell # Spell checking tools
make tests # Run all tests
make test_watch # Run tests in watch mode
make lint # Run linter
make format # Format code
make spell_check # Check spelling
make clean # Remove all generated files
Ensure you have AWS credentials configured using one of these methods:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "Statement1",
"Effect": "Allow",
"Action": [
"bedrock:CreateSession",
"bedrock:GetSession",
"bedrock:UpdateSession",
"bedrock:DeleteSession",
"bedrock:EndSession",
"bedrock:ListSessions",
"bedrock:CreateInvocation",
"bedrock:ListInvocations",
"bedrock:PutInvocationStep",
"bedrock:GetInvocationStep",
"bedrock:ListInvocationSteps"
],
"Resource": [
"*"
]
},
{
"Effect": "Allow",
"Action": [
"kms:Decrypt",
"kms:Encrypt",
"kms:GenerateDataKey",
"kms:DescribeKey"
],
"Resource": "arn:aws:kms:{region}:{account}:key/{kms-key-id}"
},
{
"Effect": "Allow",
"Action": [
"bedrock:TagResource",
"bedrock:UntagResource",
"bedrock:ListTagsForResource"
],
"Resource": "arn:aws:bedrock:{region}:{account}:session/*"
}
]
}
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A LangChain checkpointer implementation that uses Bedrock Session Management Service to enable stateful and resumable LangGraph agents.
We found that langgraph-checkpoint-aws demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.