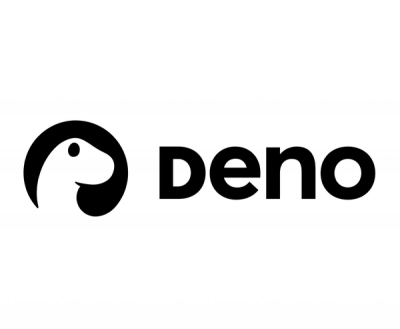
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This is the Python API for the HID-IO layouts repository. By default, the API will download the latest version of the layouts git repository to use that as a cache.
The purpose of this API is to acquire and merge the JSON HID layouts. With some additional helpers to deal with string composition.
layouts is also available on PyPi.
pip install layouts
Some basic usage examples.
GitHub Cache
import layouts
mgr = layouts.Layouts()
print(mgr.list_layouts()
Local Cache
import layouts
layout_dir = "/tmp/mylayouts/layouts"
mgr = layouts.Layouts(layout_path=layout_dir)
print(mgr.list_layouts()
import layouts
mgr = layouts.Layouts()
layout = mgr.get_layout('default')
print(layout.name()) # Name of merged layout
print(layout.json()) # Fully merged JSON dict
print(layout.locale()) # Tuple (<USB HID locale code>, <name>)
import layouts
mgr = layouts.Layouts()
layout = mgr.get_layout('default')
input_str = "Hello World!"
print(layout.compose(input_str))
# Only use code clears when necessary (blank USB packet)
print(layout.compose(input_str, minimal_clears=True))
import layouts
import layouts.emitter
mgr = layouts.Layouts()
layout = mgr.get_layout('default')
# Returns a list of list of tuples
# Each type of code has a pre-defined (configurable) prefix
# [<keyboard codes>, <led codes>, <system control codes>, <consumer codes>]
# (<name>, <code>)
# Useful for:
# #define KEY_A 0x04
print(layouts.emitter.basic_c_defines(layout))
FAQs
Python API for HID-IO HID Layouts Repository
We found that layouts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.