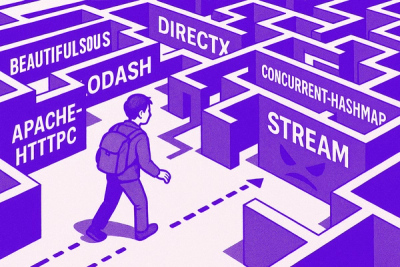
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
logging-bullet-train
Advanced tools
A bullet-train style Python logging utility that enhances your logging output with colorful and emoji-enhanced messages for better readability and quicker debugging.
You can install logging-bullet-train
using pip
:
pip install logging-bullet-train
For development purposes, use Poetry to install all dependencies:
poetry install
Here's a simple example to get you started:
import logging
import logging_bullet_train
# Set up the logger
logger = logging_bullet_train.set_logger("my_logger", level=logging.DEBUG)
# Log messages with different severity levels
logger.debug("This is a debug message") # 🔎 DEBUG
logger.info("This is an info message") # 💡 INFO
logger.warning("This is a warning message") # ⭐ WARNING
logger.error("This is an error message") # 🚨 ERROR
logger.critical("This is a critical message") # 🔥 CRITICAL
logger.log(1, "notset message") # 🔘 UNKNOWN
Sample Output:
2024-12-21T08:08:12+00:00 🔎 DEBUG my_logger:12 This is a debug message
2024-12-21T08:08:12+00:00 💡 INFO my_logger:13 This is an info message
2024-12-21T08:08:12+00:00 ⭐ WARNING my_logger:14 This is a warning message
2024-12-21T08:08:12+00:00 🚨 ERROR my_logger:15 This is an error message
2024-12-21T08:08:12+00:00 🔥 CRITICAL my_logger:16 This is a critical message
2024-12-21T08:08:12+00:00 🔘 UNKNOWN my_logger:17 notset message
By default, the logger uses a set of emojis defined in the default
theme:
Additional themes such as fruit
, weather
, and night
are available in the source code. To switch themes, you can modify the formatter to use a different emoji mapping. For example:
from logging_bullet_train import level_emoji_fruit, BulletTrainFormatter, set_logger
class FruitBulletTrainFormatter(BulletTrainFormatter):
def format(self, record):
# Override emoji selection to use fruit theme
level = record.levelno
emoji = level_emoji_fruit.get(level, level_emoji_fruit[logging_bullet_train.LOGGING_UNKNOWN])
record.levelname = f"{emoji} {record.levelname}"
return super().format(record)
# Set up the logger with the custom formatter
logger = logging.getLogger("fruit_logger")
handler = logging.StreamHandler()
handler.setFormatter(FruitBulletTrainFormatter())
logger.addHandler(handler)
logger.setLevel(logging.DEBUG)
logger.info("This info message uses fruit emojis")
The logger supports customization of colors and formatting. You can adjust color schemes or extend the formatter to better suit your needs by modifying the color dictionaries or overriding the BulletTrainFormatter
methods.
from logging_bullet_train import set_logger, wrap_text, Fore, Back
# Set up a custom logger
custom_logger = set_logger("custom_logger", level=logging.DEBUG)
# Use custom color styling in your messages if needed
message = wrap_text("Custom styled message", fg=Fore.CYAN, bg=Back.BLACK)
custom_logger.info(message)
This project is licensed under the MIT License. See the LICENSE file for details.
FAQs
A bullet-train style Python logging utils.
We found that logging-bullet-train demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.