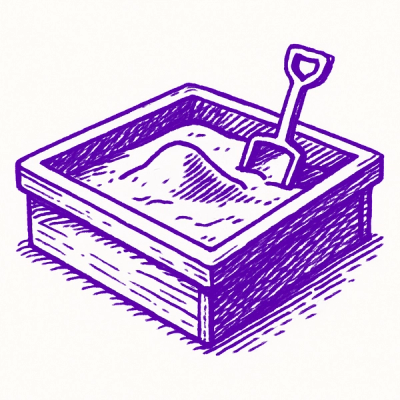
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
An agentic AI assistant built on top of the Llama language model, designed to provide intelligent assistance through a robust function calling system. It offers seamless integration of chat capabilities with file operations and memory management.
Lucius Assistant is an agentic AI assistant built on top of the Llama language model, designed to provide intelligent assistance through a robust function calling system. It offers seamless integration of chat capabilities with file operations and memory management.
pip install luciusassistant
from luciusassistant import LuciusAssistant, get_builtin_function_calls
# Initialize Lucius with built-in functions
lucius = LuciusAssistant()
# Contains a built-in function to list the current directory and read files
lucius.set_function_calls(get_builtin_function_calls())
def print_response(mode: int, delta: str, text: str):
if mode == 0:
print("[Lucius]:")
elif mode == 1:
print(delta, end="", flush=True)
elif mode == 2:
print("")
lucius.engine.stream_event.on(print_response)
# Chat with Lucius
response = lucius.chat("Hello, can you take a look at the current project?")
from luciusassistant import LuciusAssistant, get_builtin_function_calls
# Initialize with built-in functions
lucius = LuciusAssistant()
lucius.set_function_calls(get_builtin_function_calls())
# List directory contents
lucius.chat("List the contents of the current directory")
# Read a file
lucius.chat("Read the contents of config.json")
# Write to a file
lucius.chat("Create a new file named example.txt with 'Hello World' content")
from luciusassistant import LuciusAssistant, get_builtin_function_calls
# Initialize with built-in functions
lucius = LuciusAssistant()
lucius.set_function_calls(get_builtin_function_calls()) # Contains a built-in function to store and retrieve memories
# Store a memory
lucius.chat("Remember this phone number for support: 1-800-SUPPORT")
# Long conversation later...
# Search memories
lucius.chat("Find any stored support contact information")
# Lucius finds the support phone number memory
# Remove a memory
lucius.chat("Remove that memory")
You can extend Lucius's capabilities by creating custom functions:
from luciusassistant import LuciusAssistant, FunctionCall, get_builtin_function_calls
# Create a custom function
class WeatherFunctionCall(FunctionCall):
def __init__(self):
super().__init__(
name="get_weather",
parameters={
"city": "Name of the city",
"country": "Country code (e.g., US, UK)"
},
description="Get the current weather for a specified city"
)
def invoke(self, city: str, country: str = "US"):
try:
# Implement your weather API call here
return f"Weather information for {city}, {country}"
except Exception as e:
return f"Failed to get weather: {str(e)}"
# Initialize Lucius with both built-in and custom functions
lucius = LuciusAssistant()
function_calls = get_builtin_function_calls()
function_calls.append(WeatherFunctionCall())
lucius.set_function_calls(function_calls) # Very impotant you call this function at the start because this will clear the conversation history
# Use the custom function
lucius.chat("What's the weather like in San Francisco?")
Lucius provides three main events you can subscribe to for monitoring the conversation and function calls:
from luciusassistant import LuciusAssistant, get_builtin_function_calls
# Initialize Lucius with built-in functions
lucius = LuciusAssistant()
lucius.set_function_calls(get_builtin_function_calls())
def handle_chat_text(mode: int, delta: str, text: str, switch: bool):
# mode: 0=stream start, 1=during stream, 2=stream end
# delta: new text generated in this iteration
# text: total accumulated text so far
# switch: True only when switching from chat to function call mode
print(f"Chat text: mode={mode}, delta={delta}, text={text}, switch={switch}")
def handle_function_calls(mode: int, delta: str, text: str, switch: bool):
# mode: 0=stream start, 1=during stream, 2=stream end
# delta: new text generated in this iteration
# text: total accumulated text so far
# switch: True only when switching from chat to function call mode
print(f"Function calls: mode={mode}, delta={delta}, text={text}, switch={switch}")
def handle_automated_response(automated_response: str):
# automated_response: the response generated by the automated function
print(f"Automated response: {automated_response}")
# Subscribe to the events
lucius.chat_text_event.on(handle_chat_text) # Triggered for each iteration normal text is generated
lucius.function_calls_text_event.on(handle_function_calls) # Triggered for each iteration of function call text is generated
lucius.automated_response_event.on(handle_automated_response) # Triggered when function calls are executed and results are returned
# Example chat that will trigger events
lucius.chat("Hello, can you take a look at the current project?")
The events system allows you to:
Each event provides different information:
The mode
parameter in handlers indicates:
0
: Stream start1
: During stream2
: Stream endThe switch
parameter indicates when Lucius switches between chat and function call modes.
Lucius Assistant is built with a modular architecture:
Contributions are welcome! Please feel free to submit a Pull Request.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
An agentic AI assistant built on top of the Llama language model, designed to provide intelligent assistance through a robust function calling system. It offers seamless integration of chat capabilities with file operations and memory management.
We found that luciusassistant demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.