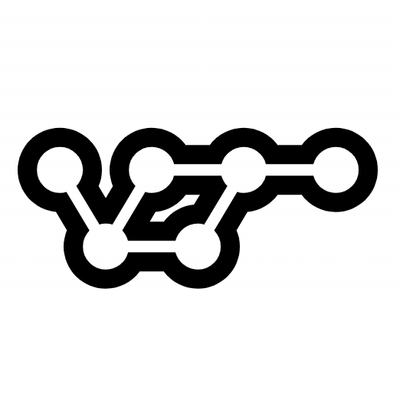
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Manage a mitmproxy service on another system over a RESTful API
pip install manageritm gunicorn
gunicorn --bind 0.0.0.0:8000 --workers 1 --log-level debug "manageritm.app:main()"
import manageritm
manageritm_addr = "localhost"
manageritm_port = "8000"
# create a manageritm client
mc = manageritm.client.ManagerITMProxyClient(f'http://{manageritm_addr}:{manageritm_port}')
proxy_details = mc.client()
print(f"proxy port: {proxy_details['port']}")
print(f"proxy webport: {proxy_details['webport']}")
# start a proxy server
mc.start()
# set your application to use the proxy
# host: "localhost"
# port: f"{proxy_details['port']}"
# do some work...
# stop the proxy server
mc.stop()
Or start a video client
flask_config_video.py
MANAGERITM_CLIENT_COMMAND = ['/opt/bin/video.sh']
Start manageritm server on port 8000.
gunicorn --bind 0.0.0.0:8000 --workers 1 --log-level debug "manageritm.app:main()"
In Python, create a client, start the ffmpeg service, stop the ffmpeg service
import manageritm
manageritm_addr = "localhost"
manageritm_port = "8000"
# create a manageritm client
mc = manageritm.client.ManagerITMCommandClient(f'http://{manageritm_addr}:{manageritm_port}')
client_details = mc.client(additional_env={
'DISPLAY_CONTAINER_NAME': 'hanet_chrome_1',
'DISPLAY_NUM': '99',
'SE_VIDEO_FOLDER': '/videos',
'FILE_NAME': 'blahh.mp4'
})
# start a proxy server
mc.start()
# recording should happening
# ...
# stop the proxy server
mc.stop()
make pyenv
make install
make server
import manageritm
manageritm_addr = "localhost"
manageritm_port = "8000"
# create a manageritm client
mc = manageritm.client.ManagerITMProxyClient(f'http://{manageritm_addr}:{manageritm_port}')
proxy_details = mc.client()
print(f"proxy port: {proxy_details['port']}")
print(f"proxy webport: {proxy_details['webport']}")
# start a proxy server
mc.start()
http://localhost:<proxy webport>
to watch the traffic# stop the proxy server
mc.stop()
To build a package for the development version:
make all
To install a copy into your local python virtualenv
make install
To run the test cases:
make test
To run the development version of the service:
make run
FAQs
Manage processes via an HTTP based API
We found that manageritm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.