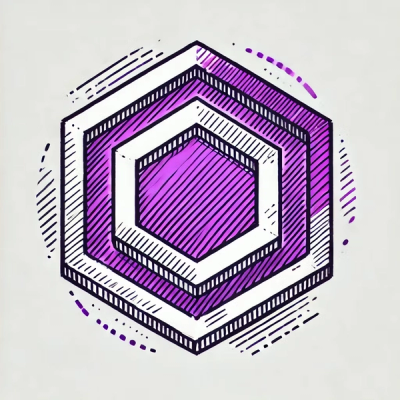
Security News
ESLint Adds Official Support for Linting HTML
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
A Python library for AI-driven mobile device automation using LLMs to control Android devices.
pip install manus_mobile
The library is designed to be LLM-agnostic, allowing you to use any LLM provider:
import asyncio
from manus_mobile import mobile_use
# Define a function to call your preferred LLM
async def call_my_llm(messages, tools=None):
# Implement your LLM calling logic here
# This is just a placeholder
return {
"role": "assistant",
"content": "I'll help automate your mobile device."
}
async def main():
# Use mobile_use with your LLM
result = await mobile_use(
task="Open the calculator app and press the number 5",
llm_function=call_my_llm
)
print(result)
if __name__ == "__main__":
asyncio.run(main())
import asyncio
import sys
from pathlib import Path
# Import mobile_use
from manus_mobile import mobile_use
# Add minion to path (if not installed via pip)
minion_path = Path('/path/to/minion')
sys.path.append(str(minion_path))
from minion.configs.config import config
from minion.providers import create_llm_provider
from minion.schema.message_types import Message
async def minion_llm_function(messages, tools=None):
"""Function to call minion LLM"""
# Get model configuration
model_name = "gpt-4o" # or any other model you prefer
llm_config = config.models.get(model_name)
if not llm_config:
raise ValueError(f"Model configuration for '{model_name}' not found")
# Create LLM provider
llm = create_llm_provider(llm_config)
# Convert messages to Minion Message format
minion_messages = [
Message(role=msg["role"], content=msg["content"])
for msg in messages
]
# Generate response
response = await llm.generate(minion_messages, tools=tools)
return {
"role": "assistant",
"content": response
}
async def main():
# Use mobile_use with Minion
result = await mobile_use(
task="Open the calculator app and press the number 5",
llm_function=minion_llm_function
)
print(result)
if __name__ == "__main__":
asyncio.run(main())
The library provides direct access to ADB functionality:
from manus_mobile import ADBClient
async def main():
# Initialize ADB client
adb = ADBClient()
# Take a screenshot
screenshot = await adb.screenshot()
# Tap on the screen
await adb.tap(500, 500)
# Type text
await adb.inputText("Hello, world!")
# Press a key
await adb.keyPress("KEYCODE_ENTER")
# Get UI hierarchy
ui = await adb.dumpUI()
if __name__ == "__main__":
asyncio.run(main())
screenshot()
: Take a screenshot of the devicetap()
: Tap at specific coordinatesswipe()
: Perform swipe gesturesinputText()
: Input textkeyPress()
: Press a specific keydumpUI()
: Get the UI hierarchy for analysisopenApp()
: Open an application by package nameMIT
FAQs
Python library for AI-driven mobile device automation
We found that manus-mobile demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.