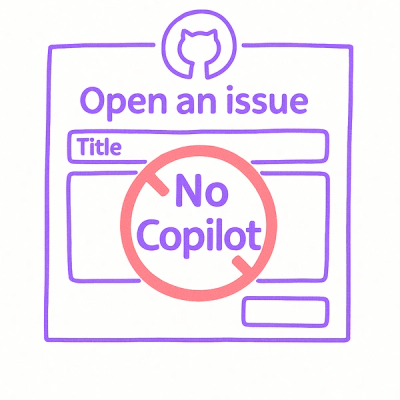
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
MATRIX Lite NFC Py is a package that allows users of varying skill levels to easily program NFC with their MATRIX Creator.
https://github.com/matrix-io/matrix-hal-nfc
To call your scripts with python 3, use
python3 YOUR_SCRIPT.py
sudo apt-get install python3-pip
python3 -m pip install --upgrade pip
sudo python3 -m pip install matrix-lite-nfc
import matrix_lite_nfc as nfc
When a read or write function completes, it will contain a status code to indicate the result. nfc.Status returns a string of what that number means.
nfc.status(/*number*/)
There can only be one instance of NFC reading. This is a hardware limitation that will not change.
A simple read that returns an object with tag data.
nfc.read.scan({
# At least 1 read options is required. Less options -> faster reading!
"info": True, # Generic information for any NFC tag
"pages": True, # All page data
"ndef": True, # A single page(faster than pages)
"page": 0, # All NDEF data
})
A non-blocking loop that passes tag data to a callback.
def read_callback(tag):
if(tag.status == 256):
print("NFC Tag Scanned!")
print(tag)
elif(tag.status == 1024):
print("Nothing Scanned!")
# Configure what you want to read
nfc.read.start({
"rate": 0.5, # Read loop speed (Seconds)
"info": True,
"pages": True,
"ndef": True,
"page": 0,
}, read_callback)
nfc.read.stop()
# Create an empty NDEF message
msg = nfc.Message()
# Add NDEF Records to message
msg.addUriRecord("https://community.matrix.one")
msg.addUriRecord("tel:+14085551212")
msg.addTextRecord("Hello World")
msg.addTextRecord("Hola Mundo", "es")
msg.addMimeMediaRecord("text/json", '{"answer": 42}')
# You then pass msg into nfc.write.message(msg)
# Get NDEF data from scanned tag
tag = nfc.read.scan({"ndef": True})
# Create & print NDEF message
msg = nfc.Message(tag.ndef.content)
print(msg.getRecords())
Writing to an NFC tag should normally be done inside the read loop.
# Create new message
msg = nfc.Message()
msg.addUriRecord("https://community.matrix.one")
# Write and then Print status codes for activation & writing
print(nfc.write.message(msg))
print(nfc.write.erase())
Be careful when writing to a page. You can accidentally lock your NFC tag!
# arg1: page you want to overwrite
# arg2: Array of numbers that represents a byte
print(nfc.write.page(25, [48,45,59,21]))
Download the repository.
git clone https://github.com/matrix-io/matrix-lite-nfc-py
Install pybind11.
sudo python3 -m pip install pybind11
Compile and install library.
sudo python3 -m pip install ./matrix-lite-nfc-py
Test your changes by importing any of the following libraries into a .py
file.
# Direct C++ bindings
import _matrix_hal_nfc as hal_nfc
# Abstractions to _matrix_hal_nfc
import matrix_lite_nfc as nfc
FAQs
A Python wrapper for MATRIX HAL NFC
We found that matrix-lite-nfc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.