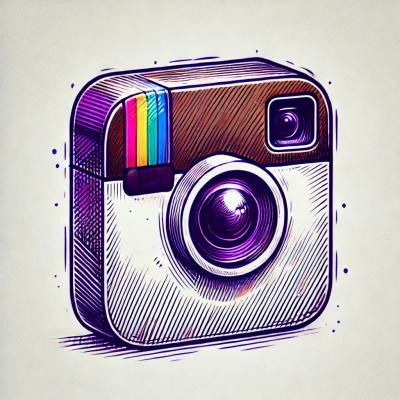
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
The mldesigner
package provide the SDK interface which work along with Azure ML Designer (drag-n-drop ML) UI experience.
Especially, the package ease the authoring experience of resources like Components
& Pipelines
:
Recommended to work with azure-ai-ml==1.13
New Features
mldesigner compile
supports to compile parallel component.mldesigner generate
supports to generate package with provided authentication.Recommended to work with azure-ai-ml==1.12
Improvements
https://azuremlschemas.azureedge.net/latest/commandComponent.schema.json
.Fixed Bugs
Recommended to work with azure-ai-ml==1.11
New Features
mldesigner compile
supports to compile with .amlignore:
mldesigner compile --source xxx.yaml --amlignore-file <path-to>/.amlignore
Recommended to work with azure-ai-ml==1.10
New Features
mldesigner compile
support v1.5 spark components.is_control=True
is not required for primitive type output.generate
package support specify version for registry component and generate
by sdk support remote url.
from mldesigner import generate
generate(
source="azureml://registries/{registry_name}/components/{component_name}/versions/0.0.8",
)
Recommended to work with azure-ai-ml==1.9.0
New Features
is_control=True
.Annotated
type as component input or component return annotation.Annotated
type in @group
.
from mldesigner import command_component, Output, Meta
from typing_extensions import Annotated
@command_component()
def my_component1(input_int: int) -> int:
return input_int
@command_component()
def my_component2(input_int: Annotated[int, Meta(description="test annotation int", min=1, max=10, default=1)],) \
-> Annotated[int, Meta(description="test annotation int")]: # using Meta class to configure more meta data
return input_int
Recommended to work with azure-ai-ml==1.7.0
Improvements
Recommended to work with azure-ai-ml==1.5.0
Improvements
mldesigner execute
:
enum
values in generate_package
.
\w
characters, e.g. empty string, -
, +
and \t
.A
and a
.curated environment
as default mldesigner component environment:
azureml://registries/azureml/environments/mldesigner-minimal/labels/latest
azureml://registries/azureml/environments/mldesigner/labels/latest
mltable
type in @dynamic
outputsFixed Bugs
Recommended to work with azure-ai-ml==1.4.0
Improvements
<4.0,>=3.7
Recommended to work with azure-ai-ml==1.3.0
New Features
@group
as component return annotation.Improvements
mldesigner execute
result.Fixed Bugs
Recommended to work with azure-ai-ml==1.2.0
Improvements
mcr.microsoft.com/azureml/openmpi4.1.0-ubuntu20.04
Fixed Bugs
**kwargs
to component funcFixed Bugs
typing_extensions
New Features
mldesigner compile
Fixed Bugs
Improvements
mode
client default value ro_mount/rw_mount
from mldesigner Input/Output
class..ipynb
.Improvements:
Fixed Bugs:
New Features
from mldesigner import command_component
@command_component
def my_component():
pass
# equals to
@command_component()
def my_component():
pass
Fixed Bugs:
New Features:
mldesigner execute --source ./components.py --name my_component inputs a=1 b=2
from mldesigner import execute
from components import my_component
node = my_component(a=1, b=2)
res = execute(node)
mldesigner execute --help
Improvements:
New Features:
from mldesigner import command_component, Input
@command_component()
def my_component(optional_param: Input(type="integer", optional=True)):
pass
Improvements:
Improvements:
New Features:
from mldesigner import command_component, Input, Output
@command_component()
def hello_world(input: Input, output: Output, param='str_param'):
print("Hello World!")
from azure.ai.ml import MLClient
client = MLClient.from_config(credential=credential)
client.components.create_or_update(hello_world)
from azure.ai.ml import dsl
@dsl.pipeline()
def my_pipeline():
node = hello_world()
return {"pipeline_output": node.outputs.output}
pipeline = my_pipeline()
client.jobs.create_or_update(pipeline)
FAQs
Azure Machine Learning Designer SDK
We found that mldesigner demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.