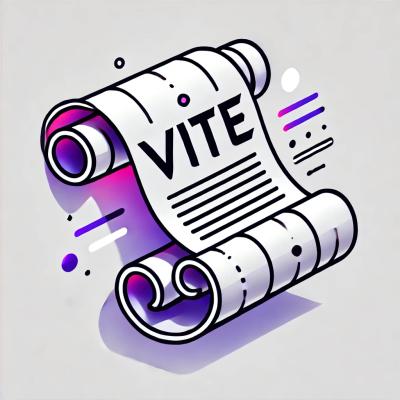
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Run compute-intensive Python functions in the cloud with a simple decorator.
Install via:
pip install nerd-mega-compute
.env
file in your project directory with:API_KEY=your_api_key_here
@cloud_compute
decorator to run your function in the cloud:from nerd_megacompute import cloud_compute
@cloud_compute(cores=8) # Specify number of CPU cores
def my_intensive_function(data):
result = process_data(data) # Your compute-intensive code
return result
result = my_intensive_function(my_data)
Test a simple addition:
from nerd_megacompute import cloud_compute
@cloud_compute(cores=2)
def add_numbers(a, b):
print("Starting simple addition...")
result = a + b
print(f"Result: {result}")
return result
print("Running a simple test...")
result = add_numbers(40, 2)
print(f"The answer is {result}")
Configure directly in code if not using a .env
file:
from nerd_megacompute import set_api_key, set_debug_mode
set_api_key('your_api_key_here')
set_debug_mode(True)
Your code can only use Python’s standard library or the following third-party libraries:
Why? This ensures compatibility and security in the cloud. Unsupported libraries may cause runtime errors.
Recommendations:
Single-threaded code: Multiple cores won’t speed up functions not designed for parallel execution.
@cloud_compute(cores=8)
def single_threaded_function(data):
return [process_item(item) for item in data]
Parallelized code: Use multi-threading or multi-processing to utilize more cores effectively.
from multiprocessing import Pool
@cloud_compute(cores=8)
def multi_core_function(data):
with Pool(8) as pool:
result = pool.map(process_item, data)
return result
This library currently supports AWS Batch with Fargate compute environments. The number of cores must be one of the following values:
cores
values to balance performance.Serialization:
Both the function and its data must be serializable (using Python’s pickle
module). Use only serializable types:
Test serialization example:
import pickle
try:
pickle.dumps(your_function_or_data)
print("Serializable!")
except pickle.PickleError:
print("Not serializable!")
Hardware Constraints: The decorator is for CPU-based tasks. For GPU tasks, consider dedicated GPU cloud services.
Internet Requirement: An active internet connection is needed during function execution.
FAQs
Run Python functions on powerful cloud servers with a simple decorator
We found that nerd-mega-compute demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.