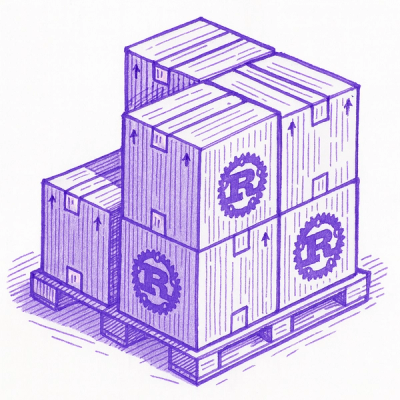
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
neutronics-material-maker
Advanced tools
A tool for making reproducible materials and standardizing use across several neutronics codes
The aim of this project is to facilitate the creation of materials for use in neutronics codes such as OpenMC, Serpent, MCNP and Fispact.
The hope is that by having this collection of materials it is easier to reuse materials across projects, use a common source with less room for user error.
One nice aspect of this material maker is the ability to change the density of a material based on isotope enrichment (e.g. inbuild lithium ceramics), temperature and pressure.
:point_right: Documentation
:point_right: Video presentation
To use the code you will need to have OpenMC installed OpenMC.
The recommended method is to install from Conda Forge which also installs all the dependencies including OpenMC.
conda create --name new_env python=3.8
conda activate new_env
conda install neutronics_material_maker -c conda-forge
Alternatively the code can be easily installed using pip. This method doesn't currently include OpenMC so that will have to be installed separately using Conda or compiled.
pip install neutronics_material_maker
To include the optional capability of calculating the density of coolants additional packages are needed (CoolProp). A slightly modified pip install is required in this case.
pip install "neutronics_material_maker[density]"
Materials for using in neutronics codes have two main aspects; a list of isotopes along with their abundance and the material density.
This code contains an internal collection of materials with their isotope abundances and densities (sometimes temperature, pressure and enrichment dependant), but you may also import your own materials collection.
Each of the materials available is stored in an internal dictionary that can be
accessed using the AvailableMaterials()
command.
import neutronics_material_maker as nmm
all_materials = nmm.AvailableMaterials()
print(all_materials.keys())
import neutronics_material_maker as nmm
my_mat = nmm.Material(
name='my_custom_material',
isotopes={'Li6':0.5, 'Li7':0.5},
density=2.01,
percent_type='ao',
density_unit='g/cm3'
)
my_mat.openmc_material
Here is an example that accesses a material from the internal collection called eurofer which has about 60 isotopes of a density of 7.78g/cm3.
import neutronics_material_maker as nmm
my_mat = nmm.Material.from_library('eurofer')
my_mat.openmc_material
For several materials within the collection the temperature and the pressure impacts the density of the material. The neutronics_material_maker adjusts the density to take temperature and the pressure into account when appropriate. Densities are calculated either by a material specific formula (for example FLiBe) or using CoolProps (for example coolants)
import neutronics_material_maker as nmm
my_mat1 = nmm.Material.from_library(name='H2O', temperature=600, pressure=15e6)
my_mat1.openmc_material
For several materials within the collection the density is adjusted when the material is enriched. For breeder blankets in fusion it is common to enrich the lithium 6 content.
Lithium ceramics used in fusion breeder blankets often contain enriched lithium-6 content. This slight change in density is accounted for by the neutronics_material_maker.
import neutronics_material_maker as nmm
my_mat2 = nmm.Material.from_library(name='Li4SiO4', enrichment=60)
my_mat2.openmc_material
Materials can also be mixed together using the from_mixture method. This
accepts a list of neutronics_material_maker.Materials
or
openmc.Material
objects along with the material fractions (fracs).
import neutronics_material_maker as nmm
my_mat1 = nmm.Material.from_library(name='Li4SiO4', packing_fraction=0.64)
my_mat2 = nmm.Material.from_library(name='Be12Ti')
my_mat3 = nmm.Material.from_mixture(materials=[my_mat1, my_mat2],
fracs=[0.4, 0.6],
percent_type='vo')
my_mat3.openmc_material
Assuming you have a JSON file saved as mat_lib.json
containing materials
defined in the same format as the
exisiting materials
then you can import this file into the package using
AddMaterialFromFile()
. Another option is to use AddMaterialFromDir()
to import a directory of json files.
import neutronics_material_maker as nmm
nmm.AddMaterialFromFile('mat_lib.json')
nmm.AvailableMaterials() # this will print the new larger list of materials
You can export to OpenMC, Serpent, MCNP and Fispact with the appropiate arguments.
import neutronics_material_maker as nmm
my_mat = nmm.Materialfrom_library('tungsten')
# openmc
my_mat.openmc_material
# mcnp - required a material_id to be set
my_mat.material_id = 1
my_mat.mcnp_material
# serpent
my_mat.serpent_material
# fispact - requires volume to be specified
my_mat.volume_in_cm3 = 1 / my_mat.density
my_mat.fispact_material
Further examples can be found in the neutronics workshop task 11 and in the Documentation
Inspired by software projects Pyne and making use of OpenMC functionality and the JSON file format and PNNL for many definitions.
FAQs
A tool for making reproducible materials and standardizing use across several neutronics codes
We found that neutronics-material-maker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.