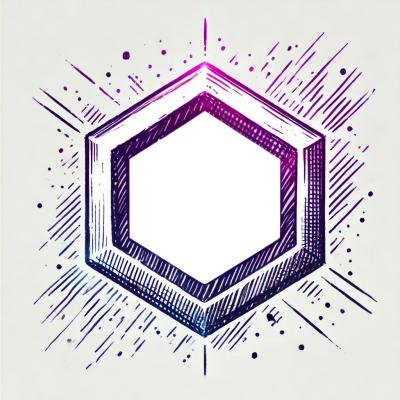
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
This is a tool for Slack API handling.
Source documentation of api is available here
pip install nftl-slack-tools
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
channel = slack.get_channels_api().create('new_order')
if channel:
print('Yupi!')
Slack API wrapper that is handling channels category
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
channels_api = slack.get_channels_api()
Lists all channels in a Slack team. Channels depends on token so if you want to list users use user token instead of SlackApi admin token
Slack API : channels.list
Args:
limit: optional page length ( default: 1000 )
cursor: optional message list cursor for pagination
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or channels :py:class:`typing.Dict`
with two keys: channels (list of channels) and cursor for pagination
when success
Create new channel with desired name
Slack API : channels.create
Args:
channel: channel name, can only contain lowercase letters, numbers,
hyphens, and underscores, and must be 21 characters or less
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or channel info as :py:class:`typing.Dict`
when success
Invite users to all the channels available in token scope except general
Slack API : Not available
Args:
users_ids: :py:class:`typing.List` of slack user ids
cursor: pagination cursor for retry etc.
invite_token: optional auth token that will overwrite SlackApi token
used for channel invitation
Returns:
boolean success status
Invite user to the channel
Slack API : channels.invite
Args:
channel: Slack channel id like CXJSD234G
user: Slack user id like UXS65F48
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or channel info as :py:class:`typing.Dict`
when success
Get channel info by channel id
Slack API : channels.info
Args:
channel_id: Slack channel id
token: optional auth token that will overwrite SlackApi token
Returns:
None if channel_id not found or channel info
as :py:class:`typing.Dict` when success
Find channel info by channel name
Slack API : Not available
Args:
channel_name: Slack channel name
token: optional auth token that will overwrite SlackApi token
Returns:
None if channel_name is not found or channel info
as :py:class:`typing.Dict` when success
Fetches history of messages and events from a channel.
Slack API : channels.history
Args:
channel: Slack channel id like CXJSF234X
count: number of messages per page
latest: End of time range
token: optional auth token that will overwrite SlackApi token
Returns:
None if error occurred or number of removed messages
Channel history cleaner Reads history and removes messages one by one
Slack API : Not available
Args:
channel_name: Slack channel name
chat: Chat api handler
Returns:
Number of removed messages
Slack API wrapper that is handling groups category
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
channels_api = slack.get_groups_api()
Lists all groups aka private channel in a Slack team. Groups depends on token so if you want to list users use user token instead of SlackApi admin token
Slack API : groups.list
Args:
limit: optional page length ( default: 1000 )
cursor: optional message list cursor for pagination
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or channels :py:class:`typing.Dict`
with two keys: groups (list of groups) and cursor for pagination
when success
Create new group with desired name
Slack API : groups.create
Args:
group: group name, can only contain lowercase letters, numbers,
hyphens, and underscores, and must be 21 characters or less
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or group info as :py:class:`typing.Dict`
when success
Invite users to all the groups available in token scope except general
Slack API : Not available
Args:
users_ids: :py:class:`typing.List` of slack user ids
cursor: pagination cursor for retry etc.
invite_token: optional auth token that will overwrite SlackApi token
used for group invitation
Returns:
boolean success status
Invite user to the group
Slack API : groups.invite
Args:
group: Slack group id like GXJSD234G
user: Slack user id like UXS65F48
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or group info as :py:class:`typing.Dict`
when success
Get group info by group id
Slack API : groups.info
Args:
group_id: Slack group id
token: optional auth token that will overwrite SlackApi token
Returns:
None if group_id not found or group info
as :py:class:`typing.Dict` when success
Find group info by group name
Slack API : Not available
Args:
group_name: Slack channel name
token: optional auth token that will overwrite SlackApi token
Returns:
None if group_name is not found or channel info
as :py:class:`typing.Dict` when success
Fetches history of messages and events from a group.
Slack API : groups.history
Args:
group: Slack group id like GXJSD234G
count: number of messages per page
latest: End of time range
token: optional auth token that will overwrite SlackApi token
Returns:
None if error occurred or number of removed messages
Group history cleaner Reads history and removes messages one by one
Slack API : Not available
Args:
group_name: Slack group name
chat: chat api handler
Returns:
Number of removed messages
Slack API wrapper that is handling users category
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
users_api = slack.get_users_api()
Gets information about the user
Slack API : users.info
Args:
user: Slack user id like UXS65F48
locale: should response contain locale info
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or user info as :py:class:`typing.Dict`
when success
Validate users name field
Slack API : This method is not documented
Args:
name: name to be validated
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or boolean validity result
Invite user to the workspace
Slack API : This method is not documented
Args:
email: account email,
firstname: account first name
lastname: account last name
channels: comma separated list of channels (id's) to be invited,
restricted: restrict to guest that can use multiple channels
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or boolean validity result
Slack API wrapper that is handling chat category
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
chat_api = slack.get_chat_api()
Deletes a message
Slack API : chat.delete
Args:
channel: Slack channel id like CXJSD234G
ts: Message id
token: optional auth token that will overwrite SlackApi token
Returns:
boolean operation status
Slack API wrapper that is handling signup category
This category is not documented in Slack doc
from nftl_slack_tools.client import SlackClient
slack = SlackClient(slack_token='xoxp-...')
chat_api = slack.get_signup_api()
Create a user with invitation code
This method is not documented in Slack doc
Args:
code: invitation code
username: user name that wil be used fo real and display name
passwd: user password to be set
locale: locale for user
tos: TOS key to be accepted
token: optional auth token that will overwrite SlackApi token
Returns:
None if data is invalid or user info as :py:class:`typing.Dict`
when success
Available here
FAQs
Tool 4 partial Slack API handling
We found that nftl-slack-tools demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.