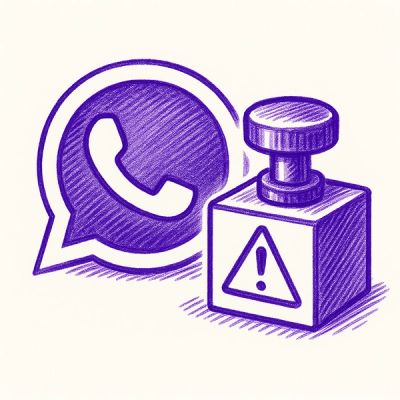
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
A Python package to interact with Nordigen API for bank account management.
A Python package to interact with the Nordigen Bank Account API, allowing users to retrieve and manage bank account details and balances.
secret_id
and secret_key
.BankAccountManager
class.NordigenAPIError
exception.Ensure Python 3.7+ is installed. To install the package, use:
pip install nordigen
To use this package, you need to obtain credentials from Nordigen, which include:
secret_id
secret_key
refresh_token
requisition_id
Follow these steps to generate the credentials:
secret_id
and secret_key
.requisition_id
.refresh_token
is retrieved when you generate an access token via the API.Once you have obtained these credentials, you can use them to connect to the API and fetch your bank account details.
The refresh_token
will expire every 30 days and the requisition_id
every 90 days and must be refreshed.
Use the create_nordigen_client
function to instantiate the Nordigen client with the required credentials.
You can pass an optional refresh token, but if not provided, the function will attempt to generate a new one using the
provided secret_id
and secret_key
.
from nordigen_account import create_nordigen_client
# Replace with your actual credentials
secret_id = "your-secret-id"
secret_key = "your-secret-key"
refresh_token = "your-refresh-token" # Optional
client, new_refresh_token = create_nordigen_client(secret_id, secret_key, refresh_token)
If your refresh token has expired, the function will automatically generate a new one.
The function will return a tuple containing the client instance and a new refresh_token
if generated, or None
if
the existing token is still valid.
Handling refresh tokens:
If a new refresh_token
is generated, make sure to update your stored credentials for subsequent API requests.
client, new_refresh_token = create_nordigen_client(secret_id, secret_key, refresh_token)
if new_refresh_token:
print("New refresh token generated:", new_refresh_token)
# Store the new token securely for future API requests
The BankAccountManager
class manages multiple bank accounts linked to a requisition ID.
When you instantiate BankAccountManager
, it automatically initializes instances of the BankAccount
class for each
linked account.
from nordigen_account import BankAccountManager
requisition_id = "your-requisition-id"
# Instantiate account manager
manager = BankAccountManager(client, requisition_id, fetch_data=True)
# Access account details
for account in manager.accounts:
print("Account ID:", account._account_id)
print("Balances:", account.balances)
By default BankAccount
data is not collected when you initialize BankAccountManager
. To optionally collect account
data, set the fetch_data
flag to True.
Two data sets are currently supported:
To access / refresh each data set use the following commands:
for account in manager.accounts:
account.update_account_data()
account.update_balance_data()
All errors related to the Nordigen API or account management are raised as a NordigenAPIError
. This exception provides:
from nordigen_account import NordigenAPIError
try:
account.update_balance_data()
except NordigenAPIError as e:
print(f"Error: {e.message}")
if e.status_code:
print(f"HTTP Status Code: {e.status_code}")
if e.response_body:
print(f"Response Body: {e.response_body}")
This unified error handling makes it easier to debug issues and ensures consistent error reporting throughout the library.
nordigen_account/
|-- nordigen_account/
| |-- __init__.py # Core functionality and class definitions
|-- setup.py # Package configuration for distribution
|-- requirements.txt # Dependencies
|-- LICENSE # License information
|-- README.md # Project documentation
|-- MANIFEST.in # Package manifest
The package requires the following dependency:
nordigen
– Python client for Nordigen API.The dependency is listed in the requirements.txt
file.
To set up the project locally:
Clone the repository:
git clone https://github.com/rahulpdev/nordigen_account.git
cd nordigen_account
Install dependencies:
pip install -r requirements.txt
Run tests or explore the package functionality.
Contributions are welcome! Please follow these steps:
This project is licensed under the MIT License - see the LICENSE file for details.
Rahul Parmar
GitHub Profile
For more details on the Nordigen API, visit their official documentation.
FAQs
A Python package to interact with Nordigen API for bank account management.
We found that nordigen-account demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.