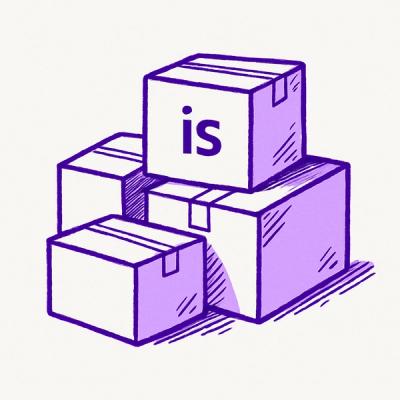
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
A flexible and modern Python ORM supporting multiple databases (MongoDB, PostgreSQL, MySQL) with both synchronous and asynchronous operations.
pip install -r requirements.txt
from odbms import Model, StringField, IntegerField, EmailField
from odbms.dbms import DBMS
# Initialize database connection
DBMS.initialize(
dbms='postgresql', # or 'mongodb', 'mysql'
host='localhost',
port=5432,
database='mydb',
username='user',
password='pass'
)
# Define your model
class User(Model):
name: str = StringField()
email: str = EmailField()
age: int = IntegerField(min_value=0)
# Create a new user
user = User(name='John Doe', email='john@example.com', age=30)
await user.save_async() # or user.save() for sync operation
# Find users
users = await User.find_async({'age': {'$gte': 25}}) # or User.find() for sync
StringField
: For text dataIntegerField
: For integer valuesFloatField
: For floating-point numbersBooleanField
: For true/false valuesDateTimeField
: For timestampsEmailField
: For email addresses with validationIDField
: For primary keys/IDsComputedField
: For dynamically computed valuesListField
: For arrays/listsDictField
: For nested documents/objects# Create
user = User(name='John', email='john@example.com')
user.save()
# Read
user = User.find_one({'email': 'john@example.com'})
users = User.find({'age': {'$gte': 25}})
all_users = User.all()
# Update
User.update({'age': {'$lt': 18}}, {'is_minor': True})
# Delete
User.remove({'status': 'inactive'})
# Aggregation
total_age = User.sum('age', {'country': 'US'})
# Create
user = User(name='Jane', email='jane@example.com')
await user.save_async()
# Read
user = await User.find_one_async({'email': 'jane@example.com'})
users = await User.find_async({'age': {'$gte': 25}})
all_users = await User.all_async()
# Update
await User.update_async({'age': {'$lt': 18}}, {'is_minor': True})
# Delete
await User.remove_async({'status': 'inactive'})
# Aggregation
total_age = await User.sum_async('age', {'country': 'US'})
class Post(Model):
title: str = StringField()
content: str = StringField()
author_id: str = IDField()
class User(Model):
name: str = StringField()
posts: List[Post] = ListField(model=Post)
# Create related records
user = User(name='John')
await user.save_async()
post = Post(title='Hello', content='World', author_id=user.id)
await post.save_async()
# Access relationships
user_posts = await user.posts # Automatically fetches related posts
Run the test suite:
pytest tests/
The test suite includes comprehensive tests for:
MIT License
FAQs
Database client for Mysql, MongoDB and Sqlite
We found that odbms demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.