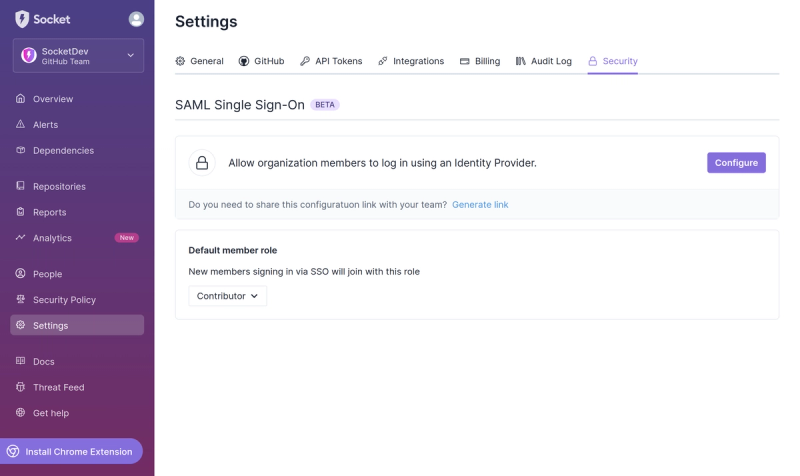
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Readme
Features:
Emphasis on API Design: Much emphasis has been put on API design and programmatic constructs in order to be able to build complex responsive webpages and websites with codebase that easy to read and maintain.
Scalable and efficient: Ofjustpy enables programmers to write scalable and efficient webpage code. It maintains only a single copy of static components, regardless of the number of connections opened. Data copying per connection and per event is minized. Even for mutable components, only a single copy of the component's static portion is maintained. Additionally, communication is efficient as it transmits only the changes (diff) to the UI to the frontend.
Composibility: Ofjustpy allows users to easily build reusable components and incorporate them into their codebase. See htmlcomponents.py and ofjustpy-components for simple to complex components build using Ofjustpy framework.
Tailwind CSS Support: Ofjustpy provides first-class and native support for Tailwind CSS constructs, enabling complex manipulation (add, remove, update classes) of the frontend UI through pure Python expressions.
Async Processing: Like its predecessor Justpy, Ofjustpy utilizes an asynchronous webserver stack that includes Starlette, ASGI, asyncio, and async/await. This architecture enables Ofjustpy to efficiently handle concurrent connections, even on single-core hardware or virtual environments, without the need for OS-level multi-threading.
Technology Stack: The tech stack of ofjustpy consists of Svelte (as the frontend UI engine), websockets (for communication between browser and server), Starlette (the async webserver), amd Tailwind (for layout and design).
See examples for a collection of basic tutorial examples of webpages build using ofjustpy.
See Basic capabilities demo for webpage showing basic html components
See Advanced capabilities demo for webpage illustrating advanced components (hierarchy navigator, pagination) in action.
The basic paradigm for building out webpages in Ofjustpy is straighforward:
If you are new to web development, then it would be helpful to go through the justpy docs and its examples on which Ofjustpy is based on. Also, pay careful attention to known corner cases and suggested workarounds mentioned in Gotchas to keep Ofjutpy engine happy
The demo example illustrates the three paradigms for building out webpages:
import ofjustpy as oj
from py_tailwind_utils import *
labels = [oj.Mutable.Label(text="mytext1", key="mylabel1"),
oj.Mutable.Label(text="mytext2", key="mylabel2"),
oj.Mutable.Label(text="mytext3", key="mylabel3"),
oj.Mutable.Label(text="mytext4", key="mylabel4"),
]
mydeck = oj.Mutable.StackD(key="mydeck",
childs=labels,
height_anchor_key="mylabel1",
twsty_tags=[W/"1/2"])
idx = 1
def on_btn_click(dbref, msg, target_of):
global idx
mydeck_shell = target_of(mydeck)
mydeck_shell.bring_to_front(labels[idx].id)
idx = (idx + 1)%4
pass
mybtn = oj.AC.Button(key="mybtn",
text="abtn",
pcp=[W/32, H/32, bg/rose/6],
on_click=on_btn_click
)
wp_endpoint = oj.create_endpoint(key="static-components",
childs = [mydeck,
mybtn
]
)
oj.add_jproute("/", wp_endpoint)
Ofjustpy, boradly, defines 2 kinds of components: static vs mutable. Static components are further classified into Passive and Active, while mutable components come in three variety: HCCMutable, HCCStatic, Mutable.
As name suggests, these do not respond to UI events and also do not undergo any changes. Ofjustpy maintains only a single copy of such components (see (see examples/static_webpages) Examples of passive components:
etc. See SHC_types.py for list of all passive components.
Active components handle events but like passive do not mutate. Active components require an additional key/id argument (see examples/input_components). Examples of active components:
and so on. See SHC_types.py for list of all active components
Mutable components can handle events and also mutate, i.e., change appearances. See examples/mutable_webpages for usage. Examples of mutable components include:
These are div components that contain mutable childrens. The div itself is not mutable (see examples/mutable_webpages/example_004.py). HCCMutable components offer minor space optimization over Mutable divs. Examples of HCCMutable components:
These are div components that itself are mutable but they contain static (passive or active) components. Examples of HCCStatic components:
See MHC_types.py for list of all HCCStatic components. jj
In addition to standard HTML components (like, Div, Span, Button, etc.), Ofjustpy offers advanced components that offer complex functionality. These are build using Tailwind CSS constructs, Svelte, and Python.
Containers to juxtapose child components
StackH, StackV, StackG, StackW : Stack components horizontally, vertically, grid, and wrap around. See examples examples/static_webpages/example_001.py,
examples/static_webpages/example_002.py for StackV, examples/static_webpages/example_004.py for StackG, examples/static_webpages/example_002.py for StackW.
StackD: Deck the items on top of each other. See examples examples/mutable_webpages/example_005.py, examples/mutable_webpages/example_008.py
Slider A component with discreet value buttons to select a value. See examples examples/mutable_webpages/example_002.py, examples/mutable_webpages/example_003.py, examples/mutable_webpages/example_006.py.
ColorSelector Component create select drop down option for all tailwind preset color values. See example examples/mutable_webpages/example_007.py
See ofjustpy-components github repo for collection of advanced components.
Ofjustpy requires that starlette app be defined in its own file lets say
"theapp.py". Then set the OJ_APP_MODULE
to set the location of the theapp.py
relative to directory from where the webserver is being launched.
export OJ_APP_MODULE="../the_app"
A sample example of theapp.py
looks as follows:
from asgi_signing_middleware import SerializedSignedCookieMiddleware
import ofjustpy as oj
csrf_middleware = Middleware(CSRFMiddleware,
secret=csrf_secret,
field_name = csrf_cookie_name)
signed_cookie_middleware = Middleware(SerializedSignedCookieMiddleware,
secret=b'a very, very secret thing',
state_attribute_name='messages',
cookie_name='my_cookie',
cookie_ttl=60 * 5,
)
auth_middleware = Middleware(AuthenticationMiddleware,
backend=BasicAuthBackend(),
on_error=lambda _, exc: PlainTextResponse("error during authentication", status_code=401)
)
app = oj.load_app([auth_middleware,
signed_cookie_middleware,
csrf_middleware
])
The default UI style of a component is determined by its type. For each component type (e.g., Button, Span), a style is defined in the snowsty.py module, which resides within the src/ofjustpy directory.
You can customize the default style by registering a style file
with Ofjustpy. To load your custom style, set the OJSTY environment variable accordingly.
Please note that the default style for a component can be overridden using the twsty_tags
directive. You can also modify the style programmatically throu TwStyCtx context expression.
Ofjustpy provides two style definitions snowsty
and un
. The un
style does not
add any styling to the components.
An example illustrate style switching:
with oj.TwStyCtx("un"):
oj.Button(..., twsty_tags = []) # Draw a with un default styling
oj.Button(..., twsty_tags = []) # use dnowsty as default styling
In Ofjustpy, the default UI style for a component is determined by its type. Each component type, such as Button or Span, has a predefined style located in the snowsty.py
module within the src/ofjustpy
directory.
To personalize the default styling, you can register a custom style file with Ofjustpy. To make use of your custom style, simply set the OJSTY
environment variable accordingly.
Please bear in mind that you have the flexibility to override the default style for a component using the twsty_tags
directive.
In addition to setting OJSTY
, you can programmatically modify the style using the TwStyCtx
context expression.
Ofjustpy offers two predefined style definitions: snowsty
and un
. The un
style, in particular, refrains from adding any additional styling to the components.
Here's an example to illustrate style switching:
with oj.TwStyCtx("un"):
oj.Button(..., twsty_tags=[]) # Draws with the 'un' default styling
oj.Button(..., twsty_tags=[]) # Uses 'snowsty' as the default styling
By following these guidelines, you can easily tailor the default styles in Ofjustpy to suit your project's aesthetic preferences.
The MountContext construct simplifies the process of mounting all the routes defined in a module. Consider the following example:
with oj.MountCtx("examples"):
with oj.MountCtx("static_webpages"):
static_webpage_module = importlib.import_module("examples.static_webpages",
)
In the above example, all the routes
defined within the examples.static_webpages will be mounted under
examples/static_webpages
path.
MountCtx enable a plug-and-play approach for adding modules and defining routes.
This means you can import the module into multiple web app codes without needing to modify the module code for each app. This flexibility simplifies the process of extending and reusing modules across different applications.
The PageBuilderCtx context is used to modify or post-process webpage endpoints defined within a module. Let's consider the following example:
def page_builder(edir):
"""
We need edir to find the example directory within which the example is located.
"""
def page_builder_inner(key, childs, **kwargs):
title = kwargs.get('title')
nav_buttons = //define a list of navigation buttons
return oj.Mutable.WebPage(key=key,
childs = [oj.HCCMutable.StackV(childs = [oj.HCCMutable.Div(childs=childs),
oj.PC.Hr(twsty_tags=[bg/green/1]),
nav_buttons],
twsty_tags= [space/y/8]
)
],
**kwargs
)
return page_builder_inner
with oj.PageBuilderCtx(page_builder("mutable_webpages")):
with oj.MountCtx("input_components"):
input_components_module = importlib.import_module("examples.input_components",
)
In the above example, the oj.PageBuilderCtx
context is used to post-process all endpoints defined within the examples.input_components
module. Specifically, it allows for the addition of navigation buttons at the bottom of each page generated by the endpoints in this module. This structured approach simplifies the task of modifying and enhancing the behavior of web pages associated with the input_components
module by applying a consistent post-processing step to all of them.
FAQs
A webdev framework that enables development of full-stack webpages and app purely in Python
We found that ofjustpy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.