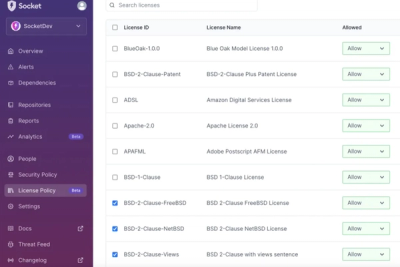
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
AWS CDK Construct that creates an Amazon API Gateway HttpApi based on a parameterized OpenAPI 3 Document.
Create an Amazon API Gateway from an OpenAPI 3 Document.
AWS CDK Construct that creates an Amazon API Gateway HttpApi based on a parameterized OpenAPI 3 Document.
pip install openapigateway
openapi.yaml:
[...]
paths:
/pets:
get:
summary: List all pets
responses:
[...]
x-amazon-apigateway-integration:
uri: "${API_LAMBDA_ARN}"
type: "AWS_PROXY"
httpMethod: "POST"
connectionType: "INTERNET"
payloadFormatVersion: "2.0"
x-amazon-apigateway-request-validator:
validateRequestBody: true
validateRequestParameters: true
[...]
open_api_stack.py:
from aws_cdk import core, aws_iam as iam, aws_lambda as _lambda
from openapigateway import OpenApiGateway
class OpenApiStack(core.Stack):
def __init__(
self, scope: core.Construct, construct_id: str, **kwargs
) -> None:
super().__init__(scope, construct_id, **kwargs)
# function that handles api request(s)
api_lambda = _lambda.Function([...])
# create api from openapi document and replace params
openapi = OpenApiGateway(
self,
"OpenAPI Gateway",
openapi_path="openapi.yaml",
param_value_dict={"API_LAMBDA_ARN": api_lambda.function_arn},
fail_on_warnings=True,
)
# grant HttpApi permission to invoke api lambda function
api_lambda.add_permission(
f"Invoke By {openapi.http_api.node.id} Permission",
principal=iam.ServicePrincipal("apigateway.amazonaws.com"),
action="lambda:InvokeFunction",
source_arn=openapi.http_api_arn,
)
# create virtualenv on MacOS and Linux
python3 -m venv .venv
# activate virtualenv
source .venv/bin/activate
To install this package, along with the tools you need to develop and publish it, run the following:
pip install -e ".[dev]"
git checkout -b feature/fooBar
)git commit -am 'Add some fooBar'
)git push origin feature/fooBar
)MIT
FAQs
AWS CDK Construct that creates an Amazon API Gateway HttpApi based on a parameterized OpenAPI 3 Document.
We found that openapigateway demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.