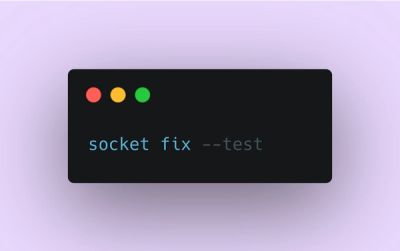
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
OxAPY is Python HTTP server library build in Rust - a fast, safe and feature-rich HTTP server implementation.
from oxapy import HttpServer, get, Router, Status, Response
@get("/")
def welcome(request):
return Response(Status.OK, "Welcome to OxAPY!")
@get("/hello/{name}")
def hello(request, name):
return Response(Status.OK, {"message": f"Hello, {name}!"})
router = Router()
router.routes([welcome, hello])
app = HttpServer(("127.0.0.1", 5555))
app.attach(router)
if __name__ == "__main__":
app.run()
def auth_middleware(request, next, **kwargs):
if "Authorization" not in request.headers:
return Status.UNAUTHORIZED
return next(request, **kwargs)
@get("/protected")
def protected(request):
return "This is protected!"
router = Router()
router.middleware(auth_middleware)
router.route(protected)
router = Router()
router.route(static_file("./static", "static"))
# Serves files from ./static directory at /static URL path
class AppState:
def __init__(self):
self.counter = 0
app = HttpServer(("127.0.0.1", 5555))
app.app_data(AppState())
@get("/count")
def handler(request):
app_data = request.app_data
app_data.counter += 1
return {"count": app_data.counter}
router = Router()
router.route(handler)
Todo:
FAQs
OxAPY is http server for python build in rust
We found that oxapy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.