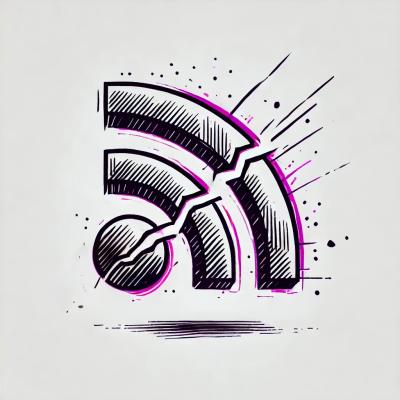
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Versatile I/O library for Python, providing easy access to audio, video, and input device capabilities.
pamiq-io is a versatile I/O library for Python, providing easy access to audio, video, and input device capabilities for interactive applications, simulations, and AI projects, made for P-AMI<Q>.
For keyboard and mouse output, you must first install inputtino.
# Install the base package
pip install pamiq-io
# Install with optional dependencies as needed
pip install pamiq-io[inputtino] # For keyboard and mouse output
pip install pamiq-io[opencv] # For video input
pip install pamiq-io[osc] # For OSC communication
pip install pamiq-io[soundcard] # For audio input/output
# For running demo scripts
sudo apt install libsndfile1
pip install pamiq-io[demo]
# Install build dependencies
sudo apt install git cmake build-essential pkg-config libevdev-dev libsndfile1
# Clone and setup
git clone https://github.com/MLShukai/pamiq-io.git
cd pamiq-io
make venv # Sets up virtual environment with all dependencies
pamiq-io includes several helpful command-line tools:
# List available video input devices
pamiq-io-show-opencv-available-input-devices
# List available audio input devices
pamiq-io-show-soundcard-available-input-devices
# List available audio output devices
pamiq-io-show-soundcard-available-output-devices
Install OBS Studio following the official installation instructions:
If the virtual camera functionality is not available after installing OBS, you may need to install v4l2loopback:
sudo apt install v4l2loopback-dkms
sudo modprobe v4l2loopback
In OBS, start the virtual camera (Tools → Start Virtual Camera)
(Optional) To find the virtual camera device, you can install v4l-utils:
sudo apt install v4l-utils
v4l2-ctl --list-devices | grep -A 1 'OBS Virtual Camera' | grep -oP '\t\K/dev.*'
A Docker configuration is provided for easy development and deployment.
# Build a basic image with required dependencies
FROM ubuntu:latest
# Install dependencies
RUN apt-get update && apt-get install -y --no-install-recommends \
python3 python3-pip \
git cmake build-essential pkg-config libevdev-dev clang \
libopencv-dev \
libsndfile1 \
pulseaudio \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
# Install pamiq-io with desired optional dependencies
RUN pip install "git+https://github.com/games-on-whales/inputtino.git#subdirectory=bindings/python&branch=stable" && \
pip install pamiq-io[inputtino,opencv,osc,soundcard,demo]
# For development, you may want to check our devcontainer configuration:
# https://github.com/MLShukai/pamiq-io/blob/main/.devcontainer/Dockerfile
When running the container, you need privileged access for hardware devices:
docker run --privileged -it your-pamiq-image
[!IMPORTANT] ⚠️ Note: The
--privileged
flag is required for hardware access to input devices.
To use audio inside Docker, you need to set up PulseAudio properly:
docker run --privileged -it \
-v ${XDG_RUNTIME_DIR}/pulse/native:${XDG_RUNTIME_DIR}/pulse/native \
-v $HOME/.config/pulse/cookie:/root/.config/pulse/cookie \
-e PULSE_SERVER=unix:${XDG_RUNTIME_DIR}/pulse/native \
-e PULSE_COOKIE=/root/.config/pulse/cookie \
your-pamiq-image
# If OpenCV is installed:
from pamiq_io.video import OpenCVVideoInput
from pamiq_io.video.input.opencv import show_video_devices
# List available video devices
show_video_devices()
# Capture from camera using default parameters
video_input = OpenCVVideoInput(camera=0)
frame = video_input.read()
# Capture with specific resolution
video_input = OpenCVVideoInput(camera=0, width=640, height=480, fps=30.0)
frame = video_input.read()
# Capture with mixed parameters (use default width, but specify height)
video_input = OpenCVVideoInput(camera=0, width=None, height=720, fps=None)
frame = video_input.read()
# If SoundCard is installed:
from pamiq_io.audio import SoundcardAudioInput, SoundcardAudioOutput
from pamiq_io.audio.input.soundcard import show_all_input_devices
from pamiq_io.audio.output.soundcard import show_all_output_devices
# List available devices
show_all_input_devices()
show_all_output_devices()
# Capture audio
audio_input = SoundcardAudioInput(sample_rate=44100, channels=2)
audio_data = audio_input.read(frame_size=1024)
# Play audio
import numpy as np
sample_rate = 44100
duration = 1.0 # seconds
t = np.linspace(0, duration, int(sample_rate * duration), endpoint=False)
sine_wave = np.sin(2 * np.pi * 440 * t).reshape(-1, 1).astype(np.float32) # 440Hz
audio_output = SoundcardAudioOutput(sample_rate=sample_rate, channels=1)
audio_output.write(sine_wave)
# If python-osc is installed:
from pamiq_io.osc import OscOutput, OscInput
# Send OSC messages
osc_output = OscOutput(host="127.0.0.1", port=9001)
osc_output.send("/test/address", 42)
# Receive OSC messages
def handler(addr, value):
print(f"Received {value} from {addr}")
osc_input = OscInput(host="127.0.0.1", port=9001)
osc_input.add_handler("/test/address", handler)
osc_input.start(blocking=False)
# If inputtino is installed:
from pamiq_io.keyboard import Key, InputtinoKeyboardOutput
# Using the InputtinoKeyboardOutput implementation
keyboard = InputtinoKeyboardOutput()
keyboard.press(Key.CTRL, Key.C) # Press Ctrl+C
keyboard.release(Key.CTRL, Key.C) # Release Ctrl+C
# If inputtino is installed:
from pamiq_io.mouse import MouseButton, InputtinoMouseOutput
# Using the InputtinoMouseOutput implementation
mouse = InputtinoMouseOutput(fps=100)
mouse.move(100, 50) # Move 100 pixels/sec right, 50 pixels/sec down
mouse.press(MouseButton.LEFT)
mouse.release(MouseButton.LEFT)
The repo includes several demo scripts to help you get started:
# Audio demos (requires pamiq-io[soundcard,demo])
python demos/soundcard_audio_input.py --list-devices
python demos/soundcard_audio_output.py --frequency 440 --duration 3
# Video demos (requires pamiq-io[opencv,demo])
python demos/opencv_video_input.py --camera 0 --output frame.png
# OSC demos (requires pamiq-io[osc])
python demos/osc_io.py
# Input simulation demos (requires pamiq-io[inputtino])
python demos/inputtino_keyboard_output.py
python demos/inputtino_mouse_output.py --radius 100 --duration 5
This project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! Please feel free to submit a Pull Request.
git checkout -b feature/amazing-feature
)make test
)git commit -m 'Add some amazing feature'
)git push origin feature/amazing-feature
)FAQs
Versatile I/O library for Python, providing easy access to audio, video, and input device capabilities.
We found that pamiq-io demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.