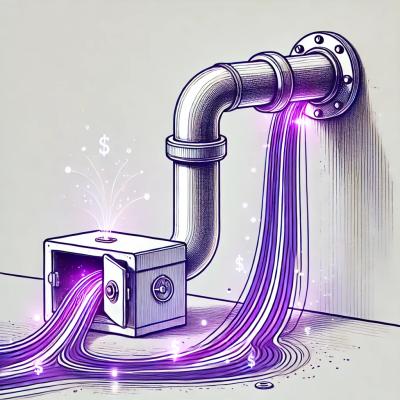
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Typed Python PostgreSQL query builder ✨
Source Code: https://github.com/yezz123/pgqb
Documentation: TBD
pgqb is a Python library for building SQL queries for PostgreSQL databases. It provides a simple and intuitive interface for constructing SQL statements using functions like delete
, insert_into
, select
, and update
. This README provides a brief overview of how to use pgqb to build queries and execute them safely with parameter binding.
You can install pgqb via pip:
pip install pgqb
from pgqb import Column, Table, select
class User(Table):
id = Column()
first = Column()
last = Column()
class Task(Table):
id = Column()
user_id = Column()
value = Column()
sql, params = (
select(User)
.from_(User)
.join(Task)
.on(Task.user_id == User.id)
.where(User.id == 1)
.order_by(Task.value.desc())
).prepare()
expected = " ".join(
[
'SELECT "user".id, "user".first, "user".last',
'FROM "user"',
'JOIN "task" ON "task".user_id = "user".id',
'WHERE "user".id = ?',
'ORDER BY "task".value DESC',
]
)
print(sql==expected)
# > True
from pgqb import Column, Table, TEXT, TIMESTAMP, UUID
class User(Table):
id = Column(UUID(), primary=True)
email = Column(TEXT(), unique=True, index=True)
first = Column(TEXT())
last = Column(TEXT())
verified_at = Column(TIMESTAMP(with_time_zone=True))
print(User.create_table())
# > CREATE TABLE IF NOT EXISTS "user" (
# "id" UUID,
# "email" TEXT NOT NULL UNIQUE,
# "first" TEXT NOT NULL,
# "last" TEXT NOT NULL,
# "verified_at" TIMESTAMP WITH TIME ZONE NOT NULL,
# PRIMARY KEY (id)
#);
# CREATE INDEX ON "user" (email);
You should create a virtual environment and activate it:
Notes: You need to have
python3.9
or higher installed.
I Use uv
to manage virtual environments, you can install it with:
# Install uv
pip install uv
# Create a virtual environment
uv venv
# Activate the virtual environment
source .venv/bin/activate
And then install the development dependencies:
# Install dependencies
uv pip install -e .[test,lint]
You can run all the tests with:
bash scripts/tests.sh
Execute the following command to apply pre-commit
formatting:
bash scripts/format.sh
Execute the following command to apply mypy
type checking:
bash scripts/lint.sh
This project is licensed under the terms of the MIT license.
FAQs
Typed Python PostgreSQL query builder using Pydantic
We found that pgqb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.