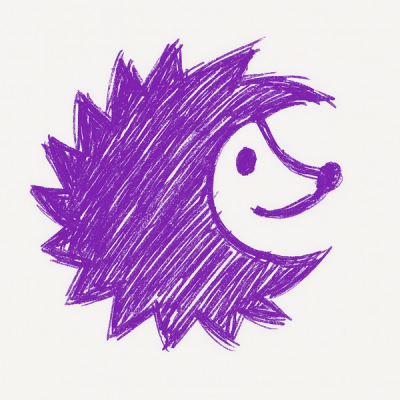
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
A asymptotically-efficient combination between a heap and a map that stores key:priority mappings. Licensed under the Apache License Version 2.0.
Operation | Description | Runtime Complexity |
---|---|---|
__getitem__(key) | Get priority of key | O(1) |
__setitem__(key, priority) | Set priority of key | O(log n) |
__delitem__(key) | Remove a key | O(log n) |
__contains__(key) | Check whether a key is contained | O(1) |
__len__() | Return the number of keys contained | O(1) |
peek() | Get lowest-priority key and its priority | O(1) |
pop() | Get and remove lowest-priority key and its priority | O(log n) |
>>> from prioritymap import PriorityMap
>>> pm = PriorityMap()
>>> pm["first"] = 1
>>> pm["second"] = 2
>>> pm["underdog"] = 5
>>> pm.peek()
('first', 1)
>>> pm["underdog"] = 0
>>> len(pm)
3
>>> pm.pop()
('underdog', 0)
>>> pm.pop()
('first', 1)
>>> pm.pop()
('second', 2)
>>> len(pm)
0
FAQs
Priority Map -- efficient heap x map
We found that prioritymap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.