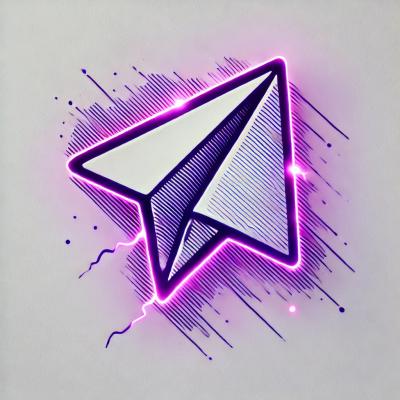
Research
npm Malware Targets Telegram Bot Developers with Persistent SSH Backdoors
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
ptest is a light test framework for Python. With ptest, you can tag test classes & test cases by decorators, execute test cases by command line, and get clear reports.
Find the latest version on github: https://github.com/KarlGong/ptest or PyPI: https://pypi.org/project/ptest
The documentation is on github wiki: https://github.com/KarlGong/ptest/wiki/documentation
The last stable release is available on PyPI and can be installed with
pip
.
$ pip install ptest
A Pycharm plugin for ptest is released. Now it is easily to run/debug ptest within the IDE using the standard run configuration. Find the latest version on JetBrains: https://plugins.jetbrains.com/plugin/7860
Firstly, create a python file: c:\folder\mytest.py
You can tag test class, test, before method, after method by adding decorator @TestClass, @Test, @BeforeMethod, @AfterMethod.
# c:\folder\mytest.py
from ptest.decorator import TestClass, Test, BeforeMethod, AfterMethod
from ptest.assertion import assert_equals, fail, assert_not_none
from ptest.plogger import preporter
from ptest import config
@TestClass(run_mode="parallel") # the test cases in this class will be executed by multiple threads
class PTestClass:
@BeforeMethod(description="Prepare test data.")
def before(self):
preporter.info("setting expected result.")
self.expected = 10
@Test(tags=["regression", "smoke"])
def test1(self):
assert_equals(10, self.expected) # pass
@Test(tags="smoke, nightly")
def test2(self):
assert_not_none(config.get_property("key")) # assert the property defined via -D<key>=<value> in cmd line
@Test(enabled=False) # won't be run
def test3(self):
fail("failed")
@AfterMethod(always_run=True, description="Clean up")
def after(self):
preporter.info("cleaning up")
Then start to execute all the testcases in module mytest.py with 2
threads. Use -w
to specify the workspace, -t
to specify the target
and -n
to specify the number of test executors(threads). In this case,
workspace is c:\folder, target is mytest and number of test
executors is 2.
Note: If you are using Windows, please confirm that %python_installation_dir%\Scripts (e.g., C:\Python35\Scripts, C:\Python37\Scripts) is added to the PATH environment variable.
$ ptest -w c:\folder -t mytest -n 2
The target can be package/module/class/method. If the target is package/module/class, all the test cases under target will be executed. For example, if you only want to execute the test test1 in this module.
$ ptest -w c:\folder -t mytest.PTestClass.test1
For more options, please use -h
.
$ ptest -h
For more code examples, please refer to the examples
folder in source
distribution or visit
https://github.com/KarlGong/ptest/tree/master/examples
For information and suggestions you can contact me at karl.gong@outlook.com
2.0.3 (compared to 2.0.2)
2.0.2 (compared to 2.0.1)
2.0.1 (compared to 2.0.0)
2.0.0 (compared to 1.9.5)
1.9.5 (compared to 1.9.4)
1.9.4 (compared to 1.9.3)
1.9.3 (compared to 1.9.2)
1.9.2 (compared to 1.9.1)
1.9.1 (compared to 1.9.0)
1.9.0 (compared to 1.8.2)
1.8.2 (compared to 1.8.1)
1.8.1 (compared to 1.8.0)
1.8.0 (compared to 1.7.7)
Support coroutine tests.
Support logging extra screenshot by preporter.
Optimize html report.
1.7.7 (compared to 1.7.6)
1.7.6 (compared to 1.7.5)
1.7.5 (compared to 1.7.4)
1.7.4 (compared to 1.7.3)
1.7.3 (compared to 1.7.2)
1.7.2 (compared to 1.7.1)
Fix @Test data provider issue in python 3.
Fix @Test timeout issue.
1.7.1 (compared to 1.7.0)
Improve performance of data provider.
Fix read property file issue.
1.7.0 (compared to 1.6.0)
Support data provider for @Test.
Fix encoding issue.
1.6.0 (compared to 1.5.3)
Add meets() in ObjSubject of assert_that assertion.
Support taking screenshots for multiple selenium webdrivers.
1.5.3 (compared to 1.5.2)
1.5.2 (compared to 1.5.1)
1.5.1 (compared to 1.4.3)
Add documentation for ptest: https://github.com/KarlGong/ptest/wiki/documentation
Add "assert_that" assertion.
Ignore the test group if no group features are used.
Support run_group for @TestClass.
Support expected_exceptions for @Test.
1.4.3 (compared to 1.4.2)
1.4.2 (compared to 1.4.1)
1.4.1 (compared to 1.4.0)
The instance variables defined in @BeforeSuite, @BeforeClass, @BeforeGroup can be accessed by other test fixtures.
Support custom args in test fixtures.
Add option (--python-paths) to specify additional python paths.
1.4.0 (compared to 1.3.2)
Support @BeforeSuite, @BeforeClass, @BeforeGroup, @AfterSuite, @AfterClass, @AfterGroup.
Support timeout for test fixtures.
Redesign the html report.
1.3.2 (compared to 1.3.1)
Add cmd line entry points for py3.
All temp data will be stored in temp folder.
1.3.1 (compared to 1.3.0)
Add examples folder.
Support declare additional arguments in test methods.
1.3.0 (compared to 1.2.2)
Support py3.
No extra package is needed to capture screenshot.
1.2.2 (compared to 1.2.1)
Support default value for config.get_property().
Add filter for test case status in html report.
1.2.1 (compared to 1.2.0)
1.2.0 (compared to 1.1.1)
Support run/debug in Pycharm via a ptest plugin.
Support filter test cases by group.
1.1.0 (compared to 1.0.4)
No extra codes are needed to support capturing screenshot for selenium test.
Add always_run attribute to @Test.
Add command option --disable-screenshot to disable taking screenshot for failed test fixture.
Support group in test class.
1.0.4 (compared to 1.0.3)
Support capture screenshot for no-selenium test.
Optimize the html report.
FAQs
light test framework for Python
We found that ptest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
Security News
pip, PDM, pip-audit, and the packaging library are already adding support for Python’s new lock file format.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.