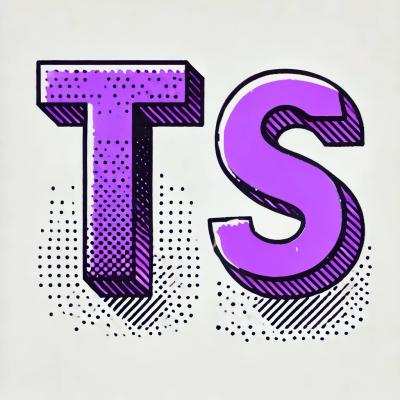
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
A tool for managing multiple 3x-ui panels at once.
This module is based on py3xui. Used dependencies:
py3xui
for connecting and managing 3xui panelspy3xui
dependencies:requests
for synchronous APIhttpx
for asynchronous APIpydantic
for modelssix
(I just cannot make py3xui work without a last version of six. This will be fixed in next versions)diskcache
for storing 3xui cookiesSupported Python Versions:
3.11
3.12
3x-ui is under development. py3xui also. I am not related with 3x-ui or py3xui. But the module supports py3xui>=0.3.4(and all version of 3x-ui that are being supported by py3xui)
pip install py_multi_3xui
from py_multi_3xui import Server
from py_multi_3xui import ServerDataManager
username = "Ben"
password = "BenLoveApples123"
host = "https://benserver.com:PORT/PATH/"
secret_token = "very_secret_token"
internet_speed = 5 # amount in gb per second.
location = "usa"
# to add a server to the db you need to create an instance of a server
server = Server(username=username, password=password, host=host, location=location, secret_token=secret_token,
internet_speed=internet_speed)
data_manager = ServerDataManager()
# after first call ServerDataManager.__init__() the servers.db will be created(if it already exists, it won't be created)
data_manager.add_server(server)
some notes:
from py_multi_3xui import ServerDataManager
host = "some_server.com:PORT/PATH/"#if you didnt specify a path it will be just "some_server.com:PORT"
manager = ServerDataManager()
manager.delete_server(host)
from py_multi_3xui import ServerDataManager
manager = ServerDataManager()
location = "usa"
best_server = await manager.choose_best_server_by_location(location)
print(best_server.__str__())
server = ...
# 1. Create client by yourself
from py3xui import Client
client = Client()
# 2. Create client using server.generate_client
from py_multi_3xui import RandomStuffGenerator as rsg
total_gb = 30 # max amount of traffic that can be used by client
inbound_id = 4 # client's inbound id
limit_ip = 0 # max amount of clients IP's. If set to zero, there is no limit
client_email = rsg.generate_email(10) # client's email. Must be unique
expiry_time = 30# expiry time in days. If set to zero, there is no limit
up = 0# a limit for upload speed. If set to zero, there is no limit
down = 0# a limit for download speed. If set to zero, there is no limit
client = server.generate_client(total_gb=total_gb,
inbound_id=inbound_id,
limit_ip=limit_ip,
client_email=client_email,
expiry_time=expiry_time,
up=up,
down=down) # note, this method is static
note: For more complete info about py3xui.Client visit py3xui documentation
from py_multi_3xui import Server
from py3xui import Client
server = ...
client = ...
server.add_client(client)
from py3xui import Client
server = ...
client = await server.get_client_by_email("some_email")
#then you edit client's fields
client.up = 50
client.down = 30
#and finally update
server.update_client(client)
from py_multi_3xui import Server
from py3xui import Client
server = ...
client = ...
config = server.get_config(client)
server = ...
uuid = "some uuid"
inbound_id = 4
server.delete_client_by_uuid(client_uuid=uuid,inbound_id=inbound_id)
Please report any bugs or feature requests by opening an issue on GitHub issues
If this project was helpful for you, you may wish star it or donate ^^
UQCOKDO9dRYNe3Us8FDK2Ctz6B4fhsonaoKpK93bqneFAyJL
##Plans:
Update README.md(or add manual) Also planned to do code-review and remove six
FAQs
module for managing multiple 3x-ui panels
We found that py-multi-3xui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.