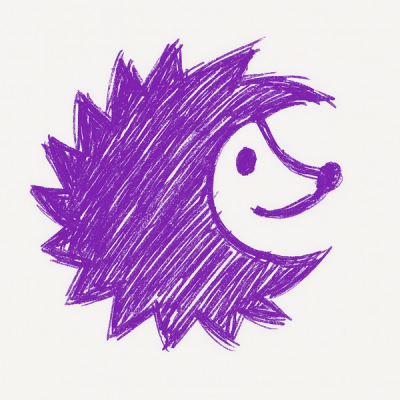
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
A simple EXR IO-library for Python that simplifies the use of OpenEXR.
pip install pyexr
Simple files
import pyexr
with pyexr.open("color.exr") as file:
file.channels # [R, G, B]
file.width # 1280
file.height # 720
file.channel_precision["R"] # pyexr.FLOAT
img = file.get() # (720,1280,3) np.float32 array
img = file.get(precision=pyexr.HALF) # (720,1280,3) np.float16 array
red = file.get("R") # (720,1280,1) np.float32 array
red = file.get("R", precision=pyexr.HALF) # (720,1280,1) np.float16 array
Fat / Multi-channel EXRs
import pyexr
with pyexr.open("multi-channel.exr") as file:
file.channels # [R, G, B, A, Variance.R, Variance.G, Variance.B]
file.width # 1280
file.height # 720
all = file.get() # (720,1280,7) np.float32 array (R,G,B,A,Var..)
var = file.get("Variance") # (720,1280,3) np.float32 array
col = file.get("default") # (720,1280,4) np.float32 array (R,G,B,A)
file.channel_map['default'] # ['R','G','B','A']
var_r = file.channel("Variance.R") # (720,1280,3) np.float32 array
import pyexr
# 'color.exr' contains R, G, B
img = pyexr.read("color.exr") # (720,1280,3) np.float32 array
img = pyexr.read("color.exr", precision=pyexr.HALF) # (720,1280,3) np.float16 array
# 'multi-channel.exr' contains R, G, B, A, Variance.R, Variance.G, Variance.B
all = pyexr.read("multi-channel.exr") # (720,1280,7) np array
col = pyexr.read("multi-channel.exr", "default") # (720,1280,4) np array
var = pyexr.read("multi-channel.exr", "Variance") # (720,1280,3) np array
col, var = pyexr.read("multi-channel.exr", ["default", "Variance"])
col, var = pyexr.read("multi-channel.exr", ["default", "Variance"], precision=pyexr.HALF)
col, var = pyexr.read("multi-channel.exr", ["default", "Variance"], precision=[pyexr.HALF, pyexr.FLOAT])
You can write a matrix to EXR without specifying channel names. Default channel names will then be used:
# columns | default |
---|---|
1 channel | Z |
2 channels | X, Y |
3 channels | R, G, B |
4 channels | R, G, B, A |
import pyexr
depth # (720,1280) np.float16 array
color # (720,180,3) np.float32 array
normal # (720,180,3) np.float32 array
pyexr.write("out.exr", depth) # one FLOAT channel: Z
pyexr.write("out.exr", color) # three FLOAT channels: R, G, B
pyexr.write("out.exr", normal,
channel_names=['X','Y','Z']) # three FLOAT channels: X, Y, Z
Writing Fat EXRs
import pyexr
depth # (720,1280) np.float16 array
color # (720,180,3) np.float32 array
variance # (720,180,3) np.float32 array
data = {'default': color, 'Depth': depth, 'Variance': variance} # default is a reserved name
pyexr.write("out.exr", data) # channels R, G, B, Depth.Z, Variance.(R,G,B)
# Full customization:
pyexr.write(
"out.exr",
data,
precision = {'default': pyexr.HALF},
channel_names = {'Depth': ['Q']}
) # channels R, G, B, Depth.Q, Variance.R, Variance.G, Variance.B
FAQs
One line EXR manipulation library
We found that pyexr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.