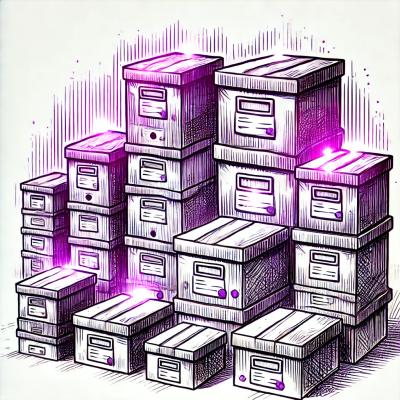
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Python bindings for REmatch, an information extraction focused regex library that uses constant delay algoirthms
Python bindings for REmatch, an information extraction focused regex library that uses constant delay algorithms.
You can install the latest release version from PyPI:
pip install pyrematch
Or you can build from the source code:
git clone git@github.com:REmatchChile/REmatch.git
cd REmatch
pip install .
Here is an example that prints all the matches using the finditer
function.
import pyrematch as REmatch
# Define the document and the REQL pattern
document = "cperez@gmail.com\npvergara@ing.uc.cl\njuansoto@uc.cl"
pattern = r"@!domain{(\w+\.)+\w+}(\n|$)"
# Create a REQL query
query = REmatch.reql(pattern)
# Execute the query and print the results
for match in query.finditer(document):
print(match)
The Query
object contains also other useful methods. To get a single match, you can use:
query.findone(document)
To find all the matches, you can use:
query.findall(document)
To find a limited number of matches, you can use:
limit = 10
query.findmany(document, limit)
To check if a match exists, you can use:
query.check(document)
You can read more about this in the PyREmatch Tutorial.
FAQs
Python bindings for REmatch, an information extraction focused regex library that uses constant delay algoirthms
We found that pyrematch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.