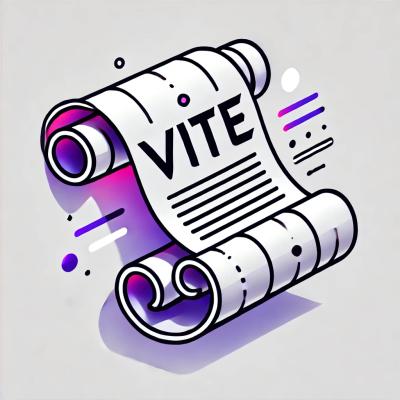
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
python-decorators-utils
Advanced tools
A collection of useful Python decorators including timing and caching
A collection of useful Python decorators including:
pip install python-decorators-utils
from python_decorators_utils import timing_decorator, cache_decorator
from python_decorators_utils import debug_decorator, execution_counter
from python_decorators_utils import retry_decorator
# Timing Decorator - Measures execution time
@timing_decorator
def slow_function():
time.sleep(1)
return "done"
# Cache Decorator - Memoizes results
@cache_decorator
def fibonacci(n):
if n < 2: return n
return fibonacci(n-1) + fibonacci(n-2)
# Debug Decorator - Logs function calls
@debug_decorator
def greet(name):
return f"Hello, {name}"
# Execution Counter - Tracks calls
@execution_counter
def counted_function():
return "I'm being counted!"
print(counted_function.get_count()) # Get number of calls
# Retry Decorator - Handles failures
@retry_decorator(max_retries=3)
def may_fail():
# Will retry up to 3 times if fails
return risky_operation()
functools.wraps
Pull requests are welcome. Please ensure tests pass before submitting.
FAQs
A collection of useful Python decorators including timing and caching
We found that python-decorators-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.