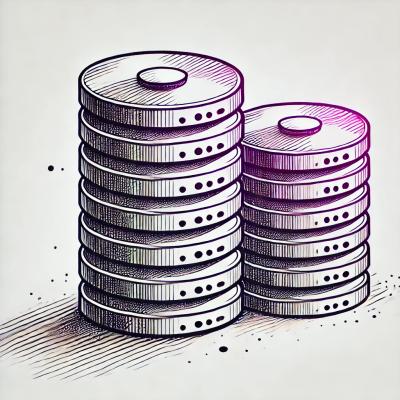
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
A modern Python library for generating QR codes with support for multiple data types, custom styling, and various output formats.
pip install qrcodex
git clone https://github.com/GamingOP69/qrcodex.git
cd qrcodex
pip install -e .
git clone https://github.com/GamingOP69/qrcodex.git
cd qrcodex
pip install -r requirements.dev.txt
pip install -e .
from qrcodex import QRCodeX
# Create a simple text QR code
qr = QRCodeX()
qr.add_data("Hello, World!")
qr.generate("output.png")
# Create a URL QR code (automatically detected)
qr = QRCodeX()
qr.add_data("https://example.com") # Automatically detected as URL
qr.generate("url.png")
from qrcodex import QRCodeX
from pathlib import Path
# Create a QR code with custom settings
qr = QRCodeX(
error_correction='H', # Highest error correction
box_size=10, # Pixel size of each module
border=4, # Border size
version=None # Auto version selection
)
# Add multiple types of data
qr.add_data("https://example.com", data_type='url')
qr.add_data(Path("image.png"), data_type='image')
qr.add_data(bytes([0x00, 0xFF]), data_type='binary')
# Generate with custom colors
qr.generate(
"output.svg",
fill_color="#FF0000", # Red QR code
back_color="white", # White background
format="svg" # SVG output
)
# High error correction for better reliability
qr = QRCodeX(error_correction='H')
qr.add_data("Important data")
qr.generate("reliable.png")
from qrcodex import QRCodeX
from pathlib import Path
# Convert image to QR code
qr = QRCodeX(error_correction='M')
qr.add_data(Path("logo.png"), data_type='image')
qr.generate("image_qr.png")
# Use existing data URI
data_uri = "data:image/png;base64,..."
qr.add_data(data_uri, data_type='image')
qr.generate("uri_qr.png")
# Create QR code with binary data
binary_data = bytes([0x00, 0xFF, 0xAA, 0x55])
qr = QRCodeX()
qr.add_data(binary_data, data_type='binary')
qr.generate("binary.png")
git clone https://github.com/GamingOP69/qrcodex.git
cd qrcodex
python -m venv venv
source venv/bin/activate # Linux/macOS
venv\Scripts\activate # Windows
pip install -r requirements.dev.txt
# Run all tests
pytest
# Run with coverage report
pytest --cov=qrcodex
# Run specific test file
pytest tests/test_generator.py
The project uses:
Run all checks:
black .
isort .
flake8 .
mypy src/qrcodex
# Build distribution packages
python -m build
# Install locally for testing
pip install -e .
This project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! Please feel free to submit a Pull Request. For major changes:
git checkout -b feature/amazing-feature
)pytest
)git commit -m 'Add amazing feature'
)git push origin feature/amazing-feature
)If you encounter any issues or have questions:
FAQs
An advanced QR code generator with multiple data type support
We found that qrcodex demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.