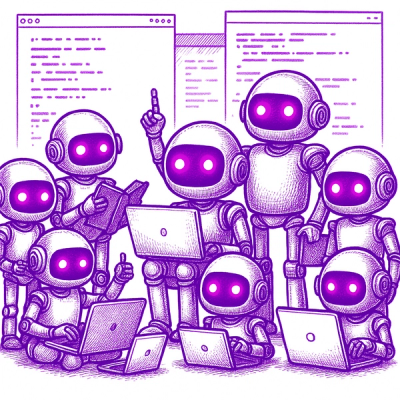
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
A Python Redis client that uses MessagePack serialization for efficient data storage and retrieval.
pip install redis-msgpack
With Django integration:
pip install redis-msgpack[django]
from redis_msgpack import RedisMsgpackClient
# Connect to Redis
client = RedisMsgpackClient(host='localhost', port=6379, db=0)
# Store Python objects directly - they will be serialized with msgpack
client.set('user:profile', {
'username': 'johndoe',
'email': 'john@example.com',
'preferences': ['dark_mode', 'notifications_on'],
'login_count': 42,
'last_login': 1647853426.78
})
# Retrieve and automatically deserialize
user_profile = client.get('user:profile')
print(user_profile['username']) # 'johndoe'
Add to your Django settings:
CACHES = {
"default": {
"BACKEND": "redis_msgpack.django_integration.RedisMsgpackCache",
"LOCATION": "redis://127.0.0.1:6379/1",
"OPTIONS": {
"CLIENT_CLASS": "redis_msgpack.client.RedisMsgpackClient",
"SERIALIZER_CLASS": "redis_msgpack.utils.MsgpackSerializer",
}
}
}
Then use Django's cache as usual:
from django.core.cache import cache
# Store complex data
cache.set('key', {'complex': 'data', 'numbers': [1, 2, 3]})
# Retrieve data
data = cache.get('key')
with client.pipeline() as pipe:
pipe.set('key1', 'value1')
pipe.set('key2', [1, 2, 3, 4])
pipe.hset('hash1', mapping={'field1': 'value1', 'field2': 'value2'})
result = pipe.execute()
pubsub = client.pubsub()
pubsub.subscribe('channel1')
# Process messages
for message in pubsub.listen():
if message['type'] == 'message':
print(f"Received: {message['data']}")
from redis_msgpack import RedisMsgpackClient
from redis_msgpack.utils import MsgpackSerializer
# Configure custom serialization options
serializer = MsgpackSerializer(use_bin_type=True, use_single_float=False)
client = RedisMsgpackClient(host='localhost', port=6379, serializer=serializer)
When compared to standard JSON serialization, redis-msgpack typically provides:
Contributions are welcome! Please feel free to submit a Pull Request.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Redis client with msgpack serialization
We found that redis-msgpack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.