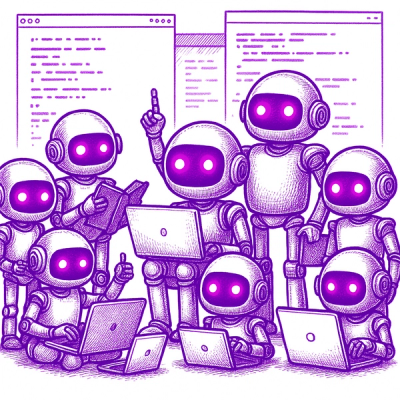
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600Ć Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Write regular expressions like poetry in Python - transform cryptic regex into elegant, readable patterns
šÆ Transform regex into poetry! Write regular expressions as elegantly as writing verses.
from repoet import op
# Traditional regex (cryptic spell)
date_regex = r"^(?P<year>\d{4})-(?P<month>\d{2})-(?P<day>\d{2})$"
# With RePoet (elegant verse)
date = op.seq(
op.begin,
op.group(op.digit * 4, name="year") + "-",
op.group(op.digit * 2, name="month") + "-",
op.group(op.digit * 2, name="day"),
op.end
)
match = date.match("2024-03-01")
print(match.group("year")) # "2024"
print(match.group("month")) # "03"
print(match.group("day")) # "01"
re
module features are supported+
, |
, *
to compose patterns naturallypip install repoet
from repoet import op
# Using operators
pattern = op.digit + op.word + op.space # \d\w+\s
pattern = op.digit | op.word # (?:\d|\w+)
pattern = op.digit * 3 # \d{3}
# Using functions
pattern = op.seq(op.digit, op.word, op.space)
pattern = op.alt(op.digit, op.word)
pattern = op.times(3)(op.digit)
# Match phone numbers with named groups
phone = op.seq(
op.maybe("+"),
op.group(op.digit * 2, "country"),
" ",
op.group(op.digit * 3, "area"),
"-",
op.group(op.digit * 4, "number")
)
match = phone.match("+86 123-4567")
print(match.group("country")) # "86"
print(match.group("number")) # "4567"
# Lookarounds
price = op.behind("$") + op.digit * 2 # (?<=\$)\d{2}
not_end = op.word + op.not_ahead(op.end) # \w+(?!$)
# Character Classes
username = op.some(op.anyof("a-zA-Z0-9_")) # [a-zA-Z0-9_]+
not_digit = op.exclude("0-9") # [^0-9]
# Quantifiers
optional = op.maybe("s") # s?
one_plus = op.some(op.letter) # \w+
any_amount = op.mightsome(op.space) # \s*
RePoet patterns support all standard re
module methods:
pattern = op.word + "@" + op.word
# All re module methods are available
pattern.match(string)
pattern.search(string)
pattern.findall(string)
pattern.finditer(string)
pattern.sub(repl, string)
pattern.split(string)
url = op.seq(
op.group(op.alt("http", "https"), "protocol"),
"://",
op.group(op.some(op.anyof("a-z0-9.-")), "domain"),
op.group(op.mightsome(op.anyof("/a-z0-9.-")), "path")
)
date = (op.digit * 4) + "-" + \
(op.digit * 2) + "-" + \
(op.digit * 2)
Contributions are welcome! Feel free to:
This project is licensed under the MIT License - see the LICENSE file for details.
āļø If you find RePoet useful, please star it on GitHub! āļø
FAQs
Write regular expressions like poetry in Python - transform cryptic regex into elegant, readable patterns
We found that repoet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.