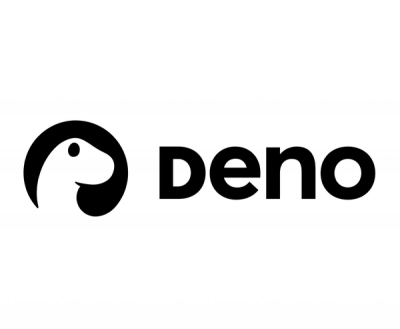
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A robust MongoDB repository pattern implementation featuring automated transaction management, type-safe operations, and comprehensive replica set support.
git clone https://github.com/yourusername/repository_mongodb.git
cd repository_mongodb
python -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
pip install -r requirements.txt
chmod +x deployment/scripts/setup-mongodb.sh
sudo ./deployment/scripts/setup-mongodb.sh
repository_mongodb/
├── repository_mongodb/
│ ├── base_model.py # Base model with MongoDB integration
│ ├── base_repository.py # Generic repository implementation
│ ├── mongo_client.py # MongoDB client configuration
│ ├── mongo_config.py # MongoDB connection settings
│ ├── transaction_management.py # Transaction context managers
│ └── transaction_metaclass.py # Automated transaction handling
├── deployment/
│ ├── docker/
│ │ ├── docker-compose.yml # MongoDB replica set configuration
│ │ └── mongodb/ # MongoDB data directory
│ └── scripts/
│ └── setup-mongodb.sh # Automated setup script
├── tests/
│ ├── test_base_repository.py # Repository tests
│ └── conftest.py # Pytest fixtures
└── README.md
The implementation uses a generic repository pattern with built-in transaction support:
class MyRepository(BaseRepository[MyModel]):
def find_by_name(self, name: str) -> Optional[MyModel]:
return self.find_by_attributes({"name": name})
Transactions are automatically handled using metaclasses:
@transactional
def transfer_funds(from_account: Account, to_account: Account, amount: float):
from_account.balance -= amount
to_account.balance += amount
account_repo.update(from_account)
account_repo.update(to_account)
The system supports flexible MongoDB configuration through environment variables:
MONGO_HOST
: MongoDB host (default: localhost)MONGO_PORT
: MongoDB port (default: 27017)MONGO_DATABASE
: Database name (default: test_database)MONGO_REPLICA_SET
: Replica set name (default: rs0)pytest tests/
Start MongoDB cluster:
cd deployment/docker
docker-compose up -d
Stop MongoDB cluster:
cd deployment/docker
docker-compose down
View logs:
docker-compose logs -f
The implementation includes comprehensive error handling:
All errors are properly logged with detailed information for debugging.
MIT License
For issues and feature requests, please use the GitHub issue tracker.
FAQs
A small library to simplify MongoDB usage with repository pattern
We found that repository-mongodb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.