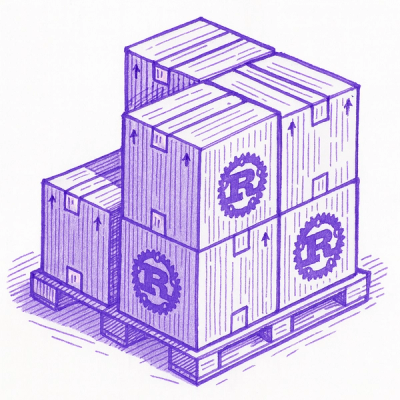
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
A Python wrapper for Rust's zip
crate that exposes a ZIP file writer with legacy ZipCrypto encryption support.
This project was primarily created using Cursor with LLMs. While it has been tested, please use it at your own risk. The code may contain unexpected behaviors or bugs.
This project is a simple wrapper over Rust's zip
crate to support writing ZIP files with ZipCrypto encryption in Python. While there are existing solutions like pyminizip, this project addresses several limitations:
This project is not intended to be a full-featured Python ZIP library (there are already many excellent options available). Instead, it focuses specifically on providing support for writing ZIP files with the legacy ZipCrypto encryption, which seems to be missing from other Python ZIP libraries.
⚠️ IMPORTANT: The ZipCrypto algorithm is considered insecure and should not be used for sensitive data. It is provided solely for compatibility with legacy systems. For secure encryption, consider using more modern alternatives.
pip install rusty-zip
from rusty_zip import ZipWriter
from io import BytesIO
# Create a new encrypted ZIP file with ZipCrypto
with ZipWriter("example.zip", password=b"mypassword") as zip_file:
# Add files to the ZIP with ZipCrypto encryption
zip_file.write_file("path/to/file.txt", "file.txt")
# Add data from memory (must be bytes)
zip_file.write_bytes(b"Hello, world!", "hello.txt")
# If you have a string, convert it to bytes first
text = "This is a string"
zip_file.write_bytes(text.encode('utf-8'), "string.txt")
# You can also use file-like objects
file_like = BytesIO()
with ZipWriter(file_like) as zip_file:
zip_file.write_file("path/to/file.txt", "file.txt")
zip_file.write_bytes(b"Hello, world!", "hello.txt")
# Get the ZIP content from the file-like object
zip_content = file_like.getvalue()
ZipWriter
ZipWriter(path_or_file_like: Union[str, BinaryIO], password: Optional[bytes] = None)
- Creates a new ZIP file. The first argument can be either a path string or a file-like object that supports binary I/O operations. If a password is provided, files will be encrypted using ZipCrypto. The password must be bytes.write_file(source_path: str, entry_name: str)
- Adds a file from disk to the ZIP archive.write_bytes(data: bytes, entry_name: str)
- Adds bytes from memory to the ZIP archive. The data must be a bytes object.close()
- Closes the ZIP file. This is automatically called when using the context manager.uv build
uv run pytest
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Python wrapper for Rust's zip crate with legacy ZipCrypto encryption support
We found that rusty-zip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.