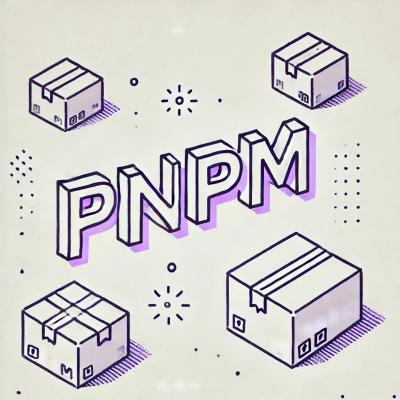
Security News
pnpm 10.12 Introduces Global Virtual Store and Expanded Version Catalogs
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
saola-graphql-client
Advanced tools
This is a GraphQL client for Python based on the ariadne-codegen
pip package. It is used to access the API at app.saola.sg.
The client provides a convenient way to interact with the Saola GraphQL API, allowing you to perform various queries and mutations related to users, connections, workspaces, chats, and sources.
To add more queries and mutations, you can create new files with the .graphql
extension in the saola_graphql_client/graphql
directory. Once you have added your new GraphQL files, run the ./saola_graphql_client/run-codegen.sh
script to generate the necessary client code.
pip install saola-graphql-client
To use the client, you can import the generated client class and use it to perform queries and mutations. Here is an example:
import asyncio
import jwt
from saola_graphql_client.graphql_client import Client as SaolaGraphQLClient
access_token = "your_access_token"
jwt_payload = jwt.decode(access_token, options={"verify_signature": False})
user_id = jwt_payload.get("hasura", {}).get("x-hasura-user-id")
client = SaolaGraphQLClient(
url="https://app.saola.sg/v1/graphql",
headers={"Authorization": f"Bearer {access_token}"},
)
async def get_and_print_current_user(client, user_id):
current_user = await client.get_user(id=user_id)
print(current_user)
asyncio.run(get_and_print_current_user(client, user_id))
Check examples/
for more examples.
Check saola_graphql_client/graphql_client/client.py for the available methods.
FAQs
A Python client for Saola's GraphQL API
We found that saola-graphql-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.