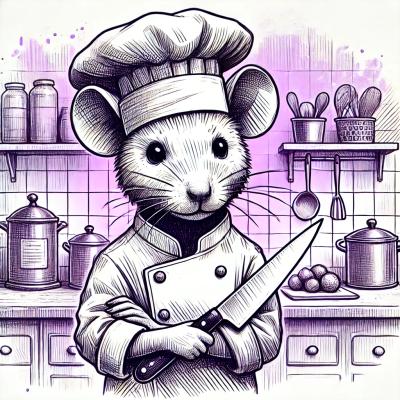
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
This repository is the home of the soon–to–be official Python API wrapper for SerpApi. This serpapi
module allows you to access search data in your Python application.
SerpApi supports Google, Google Maps, Google Shopping, Bing, Baidu, Yandex, Yahoo, eBay, App Stores, and more. Check out the documentation for a full list.
To install the serpapi
package, simply run the following command:
$ pip install serpapi
Please note that this package is separate from the legacy serpapi
module, which is available on PyPi as google-search-results
. This package is maintained by SerpApi, and is the recommended way to access the SerpApi service from Python.
Let's start by searching for Coffee on Google:
>>> import serpapi
>>> s = serpapi.search(q="Coffee", engine="google", location="Austin, Texas", hl="en", gl="us")
The s
variable now contains a SerpResults
object, which acts just like a standard dictionary, with some convenient functions added on top.
Let's print the first result:
>>> s["organic_results"][0]["link"]
'https://en.wikipedia.org/wiki/Coffee'
Let's print the title of the first result, but in a more Pythonic way:
>>> s["organic_results"][0].get("title")
'Coffee - Wikipedia'
The SerpApi.com API Documentation contains a list of all the possible parameters that can be passed to the API.
Documentation is available on Read the Docs.
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'bing',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'baidu',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'yahoo',
'p': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'youtube',
'search_query': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'walmart',
'query': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'ebay',
'_nkw': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'naver',
'query': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'home_depot',
'q': 'table',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'apple_app_store',
'term': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'duckduckgo',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google',
'q': 'coffee',
'engine': 'google',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_scholar',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_autocomplete',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_product',
'q': 'coffee',
'product_id': '4887235756540435899',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_reverse_image',
'image_url': 'https://i.imgur.com/5bGzZi7.jpg',
'max_results': '1',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_events',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_local_services',
'q': 'electrician',
'data_cid': '6745062158417646970',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_maps',
'q': 'pizza',
'll': '@40.7455096,-74.0083012,15.1z',
'type': 'search',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_jobs',
'q': 'coffee',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_play',
'q': 'kite',
'store': 'apps',
'max_results': '2',
})
import os
import serpapi
client = serpapi.Client(api_key=os.getenv("API_KEY"))
results = client.search({
'engine': 'google_images',
'tbm': 'isch',
'q': 'coffee',
})
MIT License.
Bug reports and pull requests are welcome on GitHub. Once dependencies are installed, you can run the tests with pytest
.
FAQs
The official Python client for SerpApi.com.
We found that serpapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.